相关介绍
管理系统的使用可以大大提高我们的工作效率,给我们的生活带来极大的便利,因此我们在学习编程语言的时候大多是要学习和实现一个管理系统的创建的。
学生信息管理系统是进一步推进学生学籍管理规范化、电子化控制和管理学生信息的总要举措。系统针对学校学生信息的特点以及管理中实际需要而设计,能够有效地实现学生信息管理的信息化,减轻管理人员的工作负担,高效率、规范化地管理大量的学生信息,并避免人为操作的错误和不规范行为。
接下来我会带给大家一个学生信息管理系统,该系统包括一下功能:
-
登录
-
注册
-
增添
-
删除
-
修改
-
查询
下面我们来了解一下学生信息管理系统的实现:
-
该学生信息管理系统由java+mysql数据库构成
-
主要用到的java包有:
javax.swing.JButton;
javax.swing.JFrame;
javax.swing.JLabel;
javax.swing.JPasswordField;
javax.swing.JTextField;
-
该系统设立了登录页面,设置登录及注册的相关功能,并且界面化所有功能,具备增删改查功能,并且建立了功能整个页面,将所有功能控件整合至一个页面,点击相关按钮跳转至相应的功能页面
-
运行环境:
Eclipse+JDK1.8 + MySQL
主要内容
通过java连接MySQL数据库实现学生信息管理系统,使用者能够登录并完成对信息的查询、增加、修改、删除等操作。
程序设计思维导图
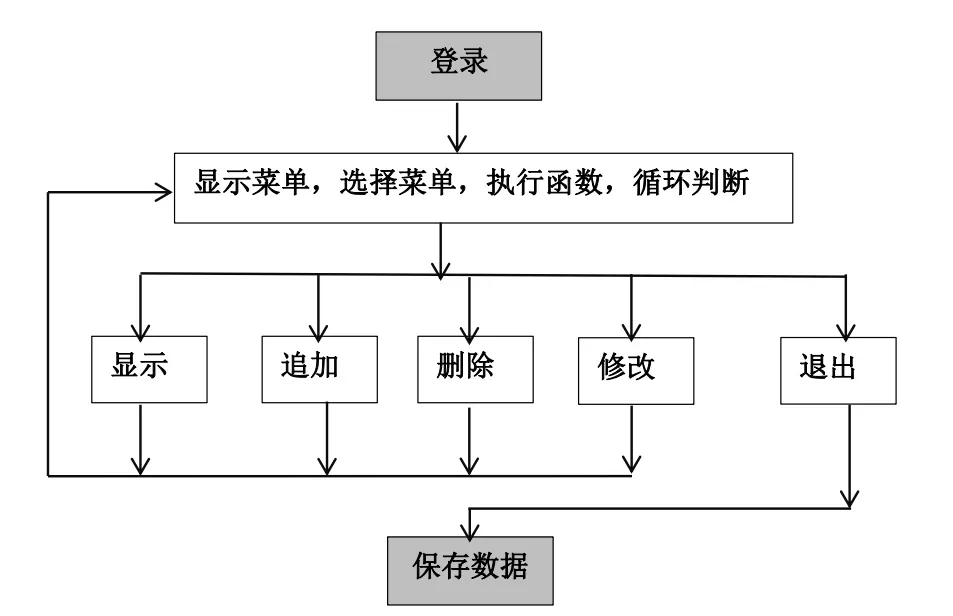
代码实现
登录页面创建
package sutdent_maniger;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class Login_Register extends JFrame{
/**
*
*/
private static final long serialVersionUID = 1L;
Login_Register() {
init();
}
//登录界面初始化
public void init() {
JFrame frame = new JFrame("登录管理系统");
frame.setLayout(null);
JLabel nameStr = new JLabel("账号:");
nameStr.setBounds(250, 200, 100, 25);
frame.add(nameStr);
JLabel passwordStr = new JLabel("密码:");
passwordStr.setBounds(250, 250, 100, 25);
frame.add(passwordStr);
JTextField userID = new JTextField();
userID.setBounds(300, 200, 150, 25);
frame.add(userID);
JPasswordField password = new JPasswordField();
password.setBounds(300, 250, 150, 25);
frame.add(password);
JButton buttonlogin = new JButton("登录");
buttonlogin.setBounds(275, 300, 70, 25);
frame.add(buttonlogin);
JButton buttonregister = new JButton("注册");
buttonregister.setBounds(375, 300, 70, 25);
frame.add(buttonregister);
frame.setBounds(400, 100, 800, 640);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
//为登录按钮添加监听器
buttonlogin.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String ID = userID.getText();
String passwd = new String (password.getPassword());
//创建一个Admin用户,把输入框中的用户名密码和提出来
Admin admin = new Admin();
admin.setID(ID);
admin.setPassword(passwd);
//登录
Login login = new Login();
login.setAdmin(admin);
if(login.JudgeAdmin()==0) {
//弹出账号或密码错误的窗口
JFrame frame = new JFrame("账号或密码错误");
frame.setLayout(null);
JButton buttonqueding = new JButton("确定");
buttonqueding.setBounds(100, 100, 70, 70);
frame.add(buttonqueding);
frame.setBounds(450, 450, 300, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
buttonqueding.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//注册页面
frame.setVisible(false);
@SuppressWarnings("unused")
Login_Register ar = new Login_Register();
}
});
//清除密码框中的信息
password.setText("");
//清除账号框中的信息
userID.setText("");
//System.out.println("登陆失败");
} else {
//弹出登录成功的窗口
JFrame frame = new JFrame("登录成功");
frame.setLayout(null);
JButton buttonqueding2 = new JButton("确定");
buttonqueding2.setBounds(100, 100, 70, 25);
frame.add(buttonqueding2);
frame.setBounds(450, 450, 300, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
buttonqueding2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//注册页面
frame.setVisible(false);
@SuppressWarnings("unused")
Window ar =new Window();
}
});
}
}
});
//为注册按钮添加监听器
buttonregister.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//注册页面
frame.setVisible(false);
@SuppressWarnings("unused")
AdminRegister ar = new AdminRegister();
}
});
}
public static void main(String []args) {
//主程序
//登录窗口
@SuppressWarnings("unused")
Login_Register login_register = new Login_Register();
}
}
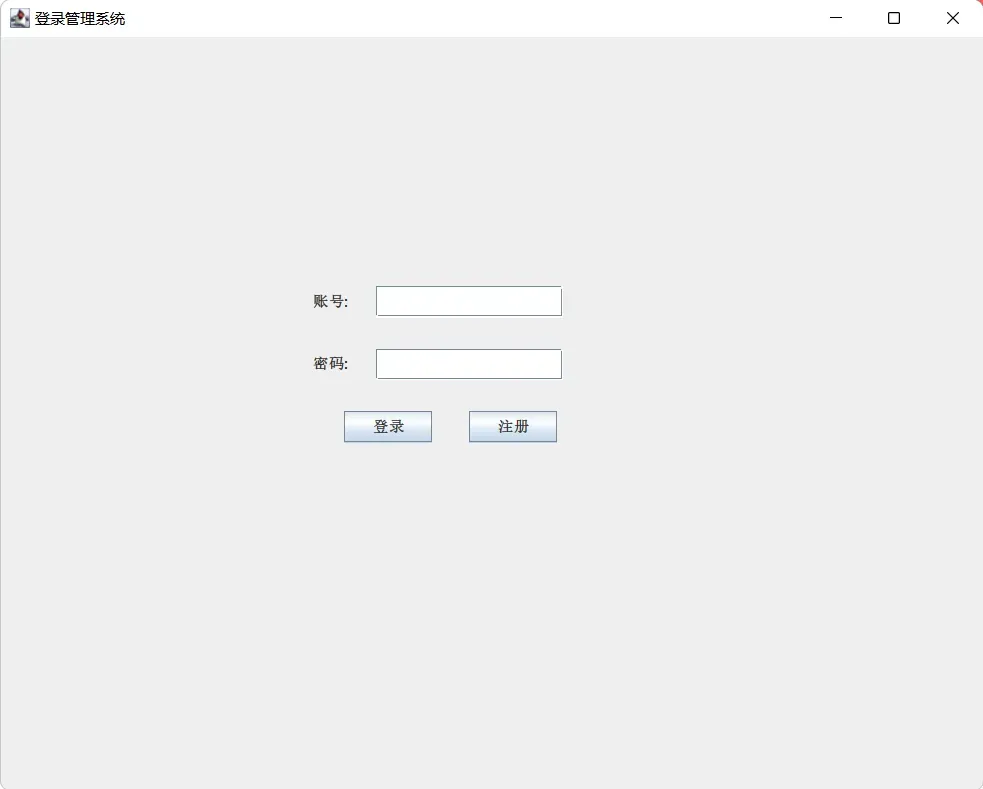
登录功能
package sutdent_maniger;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class Login {
Admin admin;
void setAdmin(Admin admin) {
this.admin=admin;
//System.out.println(this.admin.getPassword()+" " + this.admin.getID());
}
/*
* JudgeAdmin()方法
* 判断Admin的ID和密码是否正确,如果正确,显示登录成功
* 如果错误,弹出一个窗口,显示账号或密码错误
*/
private String driver = "com.mysql.cj.jdbc.Driver";
private String url = "jdbc:mysql://localhost:3306/数据库名?serverTimezone=GMT%2B8&useSSL=true"; //连接数据库(后同)
private String user = "root";
private String password = "数据库密码";
public boolean login(Admin admin) throws SQLException, ClassNotFoundException {
String sql="select * from 数据表 where id=? and password=?";
Class.forName(driver);
Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, admin.getID());
ps.setString(2, admin.getPassword());
ResultSet rs = ps.executeQuery();
int ans = 0;
if(rs.next()) {
ans = 1;
}
rs.close();
ps.close();
conn.close();
if(ans == 1) {
return true;
}
else return false;
}
int JudgeAdmin() {
try {
if(login(this.admin)) {
System.out.println("登录成功");
return 1;
}else {
return 0;
}
}catch(Exception e) {
//e.printStackTrace();
//System.out.println("!!!!!!!!!");
}
return 0;
}
}
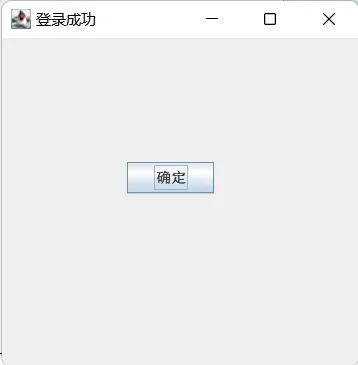
管理员注册页面
package sutdent_maniger;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
/*
* 管理员注册界面
*
*/
public class AdminRegister extends JFrame{
/**
*
*/
private static final long serialVersionUID = 1L;
AdminRegister () {
init();
}
void init() {
JFrame frame = new JFrame("注册管理员账号");
frame.setLayout(null);
JLabel nameStr = new JLabel("用户名:");
nameStr.setBounds(250, 150, 100, 25);
frame.add(nameStr);
JLabel IDStr = new JLabel("账号:");
IDStr.setBounds(250, 200, 100, 25);
frame.add(IDStr);
JLabel passwordStr = new JLabel("密码:");
passwordStr.setBounds(250, 250, 100, 25);
frame.add(passwordStr);
JLabel confrimStr = new JLabel("确认密码:");
confrimStr.setBounds(250, 300, 100, 30);
frame.add(confrimStr);
JTextField userName = new JTextField();
userName.setBounds(320, 150, 150, 25);
frame.add(userName);
JTextField userID = new JTextField();
userID.setBounds(320, 200, 150, 25);
frame.add(userID);
JPasswordField password = new JPasswordField();
password.setBounds(320, 250, 150, 25);
frame.add(password);
JPasswordField confrimPassword = new JPasswordField();
confrimPassword.setBounds(320, 300, 150, 25);
frame.add(confrimPassword);
JButton buttonregister = new JButton("注册");
buttonregister.setBounds(350, 350, 70, 25);
frame.add(buttonregister);
frame.setBounds(400, 100, 800, 640);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
//为注册按钮增加监听器
buttonregister.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String name = userName.getText();
String ID = userID.getText();
String passwd = new String (password.getPassword());
String confrimpasswd = new String (confrimPassword.getPassword());
//创建Register类
Register register = new Register();
register.setID(ID);
register.setName(name);
register.setPassword(passwd);
register.setconfirmpasswd(confrimpasswd);
//如果注册成功,返回登录界面
try {
if(register.JudgeRegister()) {
frame.setVisible(false);
@SuppressWarnings("unused")
Login_Register login_register = new Login_Register();
}
} catch (SQLException e1) {
// TODO Auto-generated catch block
//e1.printStackTrace();
} catch (ClassNotFoundException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
}
}
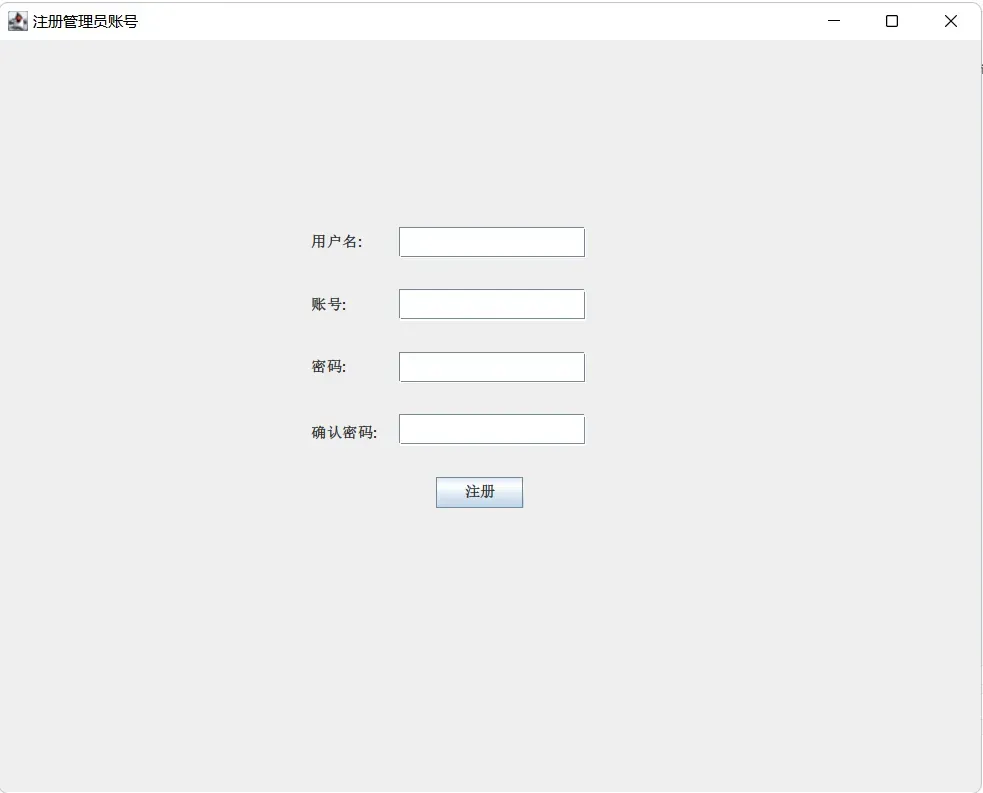
注册功能
package sutdent_maniger;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.swing.JOptionPane;
public class Register {
String name;
String ID;
String password;
String confirmpassword;
private String driver = "com.mysql.cj.jdbc.Driver";
private String url = "jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true";
private String user = "root";
private String sqlpassword = "数据库密码";
void setName(String name) {
this.name = name;
}
void setID(String ID) {
this.ID = ID;
}
void setPassword(String password) {
this.password = password;
}
void setconfirmpasswd(String confirmpassword) {
this.confirmpassword = confirmpassword;
}
//判断注册的账号是否符合规则
boolean JudgeRegister() throws SQLException, ClassNotFoundException {
if(this.name.equals("")) {
JOptionPane.showMessageDialog(null, "用户名不能为空!", "用户名", JOptionPane.ERROR_MESSAGE);
return false;
}
if(this.ID.equals("")) {
JOptionPane.showMessageDialog(null, "账号不能为空!", "账号为空", JOptionPane.ERROR_MESSAGE);
return false;
}
if(this.password.equals("")) {
JOptionPane.showMessageDialog(null, "密码不能为空!", "密码为空", JOptionPane.ERROR_MESSAGE);
return false;
}
if(!this.password.equals(this.confirmpassword)) {
JOptionPane.showMessageDialog(null, "两次输入的密码不一致!", "密码不一致", JOptionPane.ERROR_MESSAGE);
return false;
}
//符合规则,弹出注册成功的窗口,并将账号添加数据库
JOptionPane.showMessageDialog(null, "注册成功");
addAdmin();
return true;
}
//向数据库添加Admin账户
void addAdmin() throws ClassNotFoundException, SQLException {
String sql="insert into 数据表 (id, name, password) values (?,?,?)";
Class.forName(driver);
try {
Connection conn = DriverManager.getConnection(url, user, sqlpassword);
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, this.ID);
ps.setString(2, this.name);
ps.setString(3, this.password);
ps.executeUpdate();
ps.close();
conn.close();
}catch(SQLException ex) {
System.out.println("添加用户失败!");
}
}
}
功能页面创建
package sutdent_maniger;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Window extends JFrame{
/**
*
*/
private static final long serialVersionUID = 1L;
Window () {
init();
}
void init() {
JFrame jframe = new JFrame("学生管理系统") ; //window
Dimension d = new Dimension(400,300);
Point p = new Point (250,350);
jframe.setSize(d);
jframe.setLocation(p);
jframe.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jframe.setVisible(true);
JButton button1 = new JButton("添加");
JButton button2 = new JButton("修改");
JButton button3 = new JButton("查询");
JButton button4 = new JButton("删除");
JButton button5 = new JButton("浏览");
FlowLayout flow = new FlowLayout(FlowLayout.LEFT,10,10);
JPanel panel = new JPanel(flow);
panel.add(button1);
panel.add(button2);
panel.add(button3);
panel.add(button4);
panel.add(button5);
jframe.add(panel);
button1.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Add add = new Add();
}
});
button2.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Change change = new Change();
}
});
button3.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Ask ask = new Ask();
}
});
button4.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Delete delete = new Delete();
}
});
button5.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Look look = new Look();
}
});
}
}
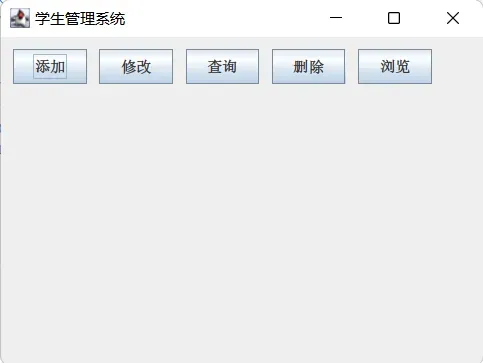
功能实现
增
package sutdent_maniger;
import java.sql.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Add extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
JLabel jlnumber = new JLabel("学号:");
JLabel jlname = new JLabel("姓名:");
JLabel jlsex = new JLabel("性别:");
JLabel jlbirthday = new JLabel("出生日期:");
JLabel jldepartment = new JLabel("学院:");
JTextField jtnumber = new JTextField("",20);
JTextField jtname = new JTextField("",20);
JTextField jtsex = new JTextField("",20);
JTextField jtbirthday = new JTextField("",20);
JTextField jtdepartment = new JTextField("",20);
JButton buttonadd = new JButton("添加");
JButton buttonreturn = new JButton("返回");
public Add() {
JPanel jpnumber = new JPanel();
JPanel jpname = new JPanel();
JPanel jpsex = new JPanel();
JPanel jpbirthday = new JPanel();
JPanel jpdepartment = new JPanel();
JPanel jpforbutton = new JPanel(new GridLayout(1,1));
jpnumber.add(jlnumber);
jpnumber.add(jtnumber);
jpname.add(jlname);
jpname.add(jtname);
jpsex.add(jlsex);
jpsex.add(jtsex);
jpbirthday.add(jlbirthday);
jpbirthday.add(jtbirthday);
jpdepartment.add(jldepartment);
jpdepartment.add(jtdepartment);
jpforbutton.add(buttonadd);
jpforbutton.add(buttonreturn);
buttonadd.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
//Add
Connection conn = null;
@SuppressWarnings("unused")
Statement stat = null;
PreparedStatement ps=null;
String sql = "INSERT INTO sqb_xueshengxinxi(number,name,sex,birthday,department) "
+ "values(?,?,?,?,?)";
try{
Class.forName("com.mysql.cj.jdbc.Driver");
System.out.println("JBDC 加载成功!");
}catch(Exception a){
System.out.println("JBDC 加载失败!");
a.printStackTrace();
}
try{
Class.forName("com.mysql.cj.jdbc.Driver");
conn=DriverManager.getConnection("jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true","root","数据库密码");
ps=conn.prepareStatement(sql);
ps.setString(1,jtnumber.getText());
ps.setString(2,jtname.getText());
ps.setString(3,jtsex.getText());
ps.setString(4,jtbirthday.getText());
ps.setString(5,jtdepartment.getText());
ps.executeUpdate();
//System.out.println("MySQL 连接成功!");
//stat = conn.createStatement();
//stat.executeUpdate(sql);
//System.out.println("插入数据成功!");
}catch (SQLException b){
b.printStackTrace();
} catch (ClassNotFoundException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}finally{
try{
conn.close();
System.out.println("MySQL 关闭成功");
}catch (SQLException c){
System.out.println("MySQL 关闭失败 ");
c.printStackTrace();
}
}
}
});
buttonreturn.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Window window = new Window();
}
});
this.setTitle("添加学生信息");
this.setLayout(new GridLayout(9,1));
this.add(jpnumber);
this.add(jpname);
this.add(jpsex);
this.add(jpbirthday);
this.add(jpdepartment);
this.add(jpforbutton);
this.setLocation(400,300);
this.setSize(350,300);
this.setVisible(true);
}
}
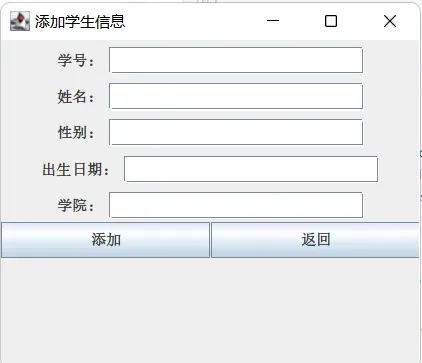
删
package sutdent_maniger;
import java.sql.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Delete extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
JLabel jlnumber = new JLabel("学号:");
JTextField jtnumber = new JTextField("",20);
JButton buttondelete = new JButton("删除");
JButton buttonreturn = new JButton("返回");
public Delete() {
JPanel jpnumber = new JPanel();
JPanel jpforbutton = new JPanel(new GridLayout(1,1));
jpnumber.add(jlnumber);
jpnumber.add(jtnumber);
jpforbutton.add(buttondelete);
jpforbutton.add(buttonreturn);
buttondelete.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
String number = jtnumber.getText();
Connection conn = null;
@SuppressWarnings("unused")
ResultSet res = null;
Statement stat = null;
String sql = "DELETE FROM sqb_xueshengxinxi WHERE number='"+number+"'";
try{
Class.forName("com.mysql.cj.jdbc.Driver");
}catch(Exception a){
a.printStackTrace();
}
try{
conn=DriverManager.getConnection("jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true","root","数据库密码");
stat = conn.createStatement();
stat.executeUpdate(sql);
}catch(SQLException h){
h.printStackTrace();
}finally{
try{
conn.close();
System.out.println("close success!");
}catch(SQLException j){
System.out.println("close go die!");
j.printStackTrace();
}
}
}
});
buttonreturn.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Window window = new Window();
}
});
this.setTitle("删除学生信息");
this.setLayout(new GridLayout(9,1));
this.add(jpnumber);
this.add(jpforbutton);
this.setLocation(400,300);
this.setSize(350,300);
this.setVisible(true);
}
}
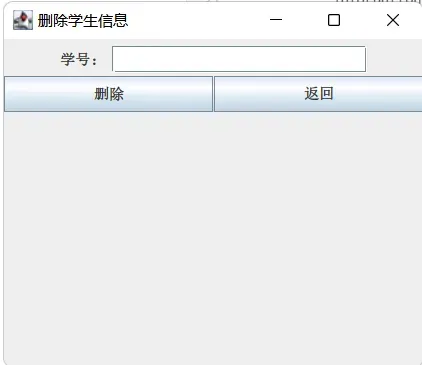
改
package sutdent_maniger;
import java.sql.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Change extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
JLabel jlnumber = new JLabel("学号:");
JLabel jlname = new JLabel("姓名:");
JLabel jlsex = new JLabel("性别:");
JLabel jlbirthday = new JLabel("出生日期:");
JLabel jldepartment = new JLabel("学院:");
JTextField jtnumber = new JTextField("",20);
JTextField jtname = new JTextField("",20);
JTextField jtsex = new JTextField("",20);
JTextField jtbirthday = new JTextField("",20);
JTextField jtdepartment = new JTextField("",20);
JButton buttonchange = new JButton("修改");
JButton buttonreturn = new JButton("返回");
public Change() {
JPanel jpnumber = new JPanel();
JPanel jpname = new JPanel();
JPanel jpsex = new JPanel();
JPanel jpbirthday = new JPanel();
JPanel jpdepartment = new JPanel();
JPanel jpforbutton = new JPanel(new GridLayout(1,1));
jpnumber.add(jlnumber);
jpnumber.add(jtnumber);
jpname.add(jlname);
jpname.add(jtname);
jpsex.add(jlsex);
jpsex.add(jtsex);
jpbirthday.add(jlbirthday);
jpbirthday.add(jtbirthday);
jpdepartment.add(jldepartment);
jpdepartment.add(jtdepartment);
jpforbutton.add(buttonchange);
jpforbutton.add(buttonreturn);
buttonchange.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
String number = jtnumber.getText();
String name = jtname.getText();
String sex = jtsex.getText();
String birthday = jtbirthday.getText();
String department = jtdepartment.getText();
Connection conn = null;
ResultSet res = null;
Statement stat = null;
String sql = "SELECT number,name,sex,birthday,department FROM 数据表;";
try{
Class.forName("com.mysql.cj.jdbc.Driver");
}catch(Exception d){
System.out.println("jdbc fall");
d.printStackTrace();
}
try{
conn=DriverManager.getConnection("jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true","root","数据库密码");
stat=conn.createStatement();
res=stat.executeQuery(sql);
while (res.next())
{
//change
if (res.getString(1).equals(jtnumber.getText()))
{
try{
Class.forName("com.mysql.cj.jdbc.Driver");
}catch(Exception d){
System.out.println("jdbc fall");
d.printStackTrace();
}
String sql2="UPDATE 数据表 SET name='"+name+"' WHERE number='"+jtnumber.getText()+"'";
String sql3="UPDATE 数据表 SET sex='"+sex+"' WHERE number='"+jtnumber.getText()+"'";
String sql4="UPDATE 数据表 SET birthday='"+birthday+"' WHERE number='"+jtnumber.getText()+"'";
String sql5="UPDATE 数据表 SET department='"+department+"' WHERE number='"+jtnumber.getText()+"'";
try {
conn=DriverManager.getConnection("jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true","root","数据库密码");
stat=conn.createStatement();
stat.executeUpdate(sql2);
stat.executeUpdate(sql3);
stat.executeUpdate(sql4);
stat.executeUpdate(sql5);
} catch (SQLException g) {
// TODO Auto-generated catch block
g.printStackTrace();
}try{
stat.close();
conn.close();
}catch(SQLException ar){
ar.printStackTrace();
}
break;
}
//change end
}
}catch (SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
finally{
try{
conn.close();
}catch(SQLException ar){
ar.printStackTrace();
}
}
}
});
buttonreturn.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Window window = new Window();
}
});
this.setTitle("修改学生信息");
this.setLayout(new GridLayout(9,1));
this.add(jpnumber);
this.add(jpname);
this.add(jpsex);
this.add(jpbirthday);
this.add(jpdepartment);
this.add(jpforbutton);
this.setLocation(400,300);
this.setSize(350,300);
this.setVisible(true);
}
}
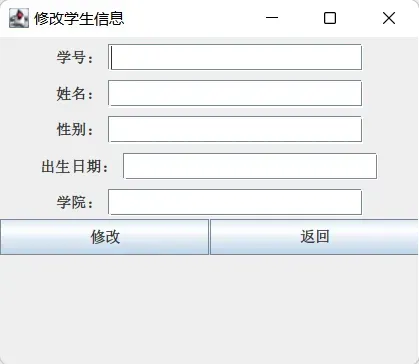
查
package sutdent_maniger;
import java.sql.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Ask extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
JLabel jlnumber = new JLabel("学号:");
JLabel jlname = new JLabel("姓名:");
JLabel jlsex = new JLabel("性别:");
JLabel jlbirthday = new JLabel("出生日期:");
JLabel jldepartment = new JLabel("学院:");
JTextField jtnumber = new JTextField("",20);
JLabel jname = new JLabel();
JLabel jsex = new JLabel();
JLabel jbirthday = new JLabel();
JLabel jdepartment = new JLabel();
JButton buttonask = new JButton("查询");
JButton buttonreturn = new JButton("返回");
public Ask() {
JPanel jpnumber = new JPanel();
JPanel jpname = new JPanel();
JPanel jpsex = new JPanel();
JPanel jpbirthday = new JPanel();
JPanel jpdepartment = new JPanel();
JPanel jpforbutton = new JPanel(new GridLayout(1,1));
jpnumber.add(jlnumber);
jpnumber.add(jtnumber);
jpname.add(jlname);
jpname.add(jname);
jpsex.add(jlsex);
jpsex.add(jsex);
jpbirthday.add(jlbirthday);
jpbirthday.add(jbirthday);
jpdepartment.add(jldepartment);
jpdepartment.add(jdepartment);
jpforbutton.add(buttonask);
jpforbutton.add(buttonreturn);
buttonask.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
Connection conn = null;
ResultSet res = null;
Statement stat = null;
String sql = "SELECT number,name,sex,birthday,department FROM 数据表;";
try{
Class.forName("com.mysql.cj.jdbc.Driver");
}catch(Exception d){
System.out.println("jdbc fall");
d.printStackTrace();
}
try{
conn=DriverManager.getConnection("jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true","root","数据库密码");
stat=conn.createStatement();
res=stat.executeQuery(sql);
while (res.next())
{
if (res.getString(1).equals(jtnumber.getText()))
{
jname.setText(res.getString(2));
jsex.setText(res.getString(3));
jbirthday.setText(res.getString(4));
jdepartment.setText(res.getString(5));
break;
}
}
}catch (SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
finally{
try{
conn.close();
}catch(SQLException ar){
ar.printStackTrace();
}
}
}
}
);
buttonreturn.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
@SuppressWarnings("unused")
Window window = new Window();
}
});
this.setTitle("查询学生信息");
this.setLayout(new GridLayout(9,1));
this.add(jpnumber);
this.add(jpname);
this.add(jpsex);
this.add(jpbirthday);
this.add(jpdepartment);
this.add(jpforbutton);
this.setLocation(400,300);
this.setSize(350,300);
this.setVisible(true);
}
}
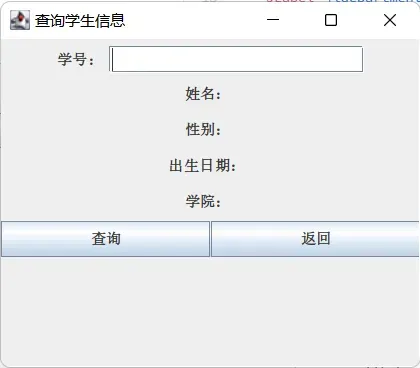
浏览所有信息
package sutdent_maniger;
import java.sql.*;
import java.awt.*;
import javax.swing.*;
import java.util.*;
public class Look extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
Connection conn = null;
PreparedStatement ps = null;
ResultSet res = null;
//JButton buttonlook = new JButton("浏览");
//JButton buttonreturn = new JButton("返回");
JTable jtable;
JScrollPane jscrollpane = new JScrollPane();
Vector<String> columnNames = null;
Vector<Vector<String>> rowData = null;
public Look() {
JPanel jpforbutton = new JPanel(new GridLayout(1,1));
columnNames = new Vector<String>();
columnNames.add("学号");
columnNames.add("姓名");
columnNames.add("性别");
columnNames.add("出生日期");
columnNames.add("学院");
rowData = new Vector<Vector<String>>();
//jpforbutton.add(buttonlook);
//jpforbutton.add(buttonreturn);
try {
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/数据库?serverTimezone=GMT%2B8&useSSL=true","root","数据库密码");
ps = conn.prepareStatement("SELECT * FROM 数据表");
res = ps.executeQuery();
while (res.next())
{
Vector<String> hang = new Vector<String>();
hang.add(res.getString(1));
hang.add(res.getString(2));
hang.add(res.getString(3));
hang.add(res.getString(4));
hang.add(res.getString(5));
rowData.add(hang);
}
System.out.println("load ok!");
}catch (Exception q){
q.printStackTrace();
System.out.println("go die");
}finally{
try{
res.close();
ps.close();
conn.close();
System.out.println("close ok");
}catch (SQLException o){
o.printStackTrace();
System.out.println("go die 2");
}
}
jtable = new JTable(rowData,columnNames);
jscrollpane = new JScrollPane(jtable);
this.add(jscrollpane);
this.setTitle("浏览学生信息");
this.setLayout(new GridLayout(2,5));
this.add(jpforbutton);
this.setLocation(300,300);
this.setSize(500,300);
this.setVisible(true);
this.setResizable(false);
}
}
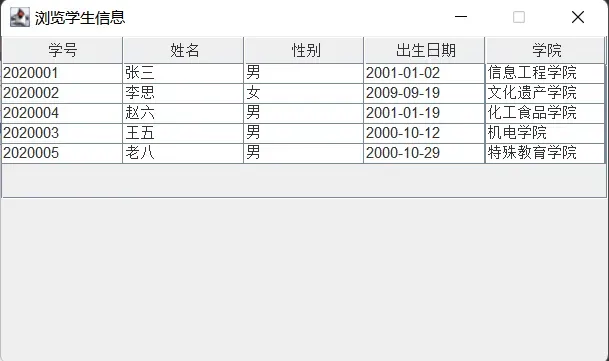
结语
利用java+MySQL实现学生信息管理系统大大提高了学生信息整理的工作效率,节约时间,节约成本,是一个很好地,非常方便的系统,对于教育工作而言是具有重要意义的。希望大家可以借助该系统可以学习到更多的编程知识,提升自我水平。
完整资源请参考
1.上传明细-CSDN创作中心
2.链接: https://pan.baidu.com/s/1pGofUyk2Vd1mOqY-ptVRBw?pwd=c2e8 提取码: c2e8 复制这段内容后打开百度网盘手机App,操作更方便哦
文章出处登录后可见!