前言
基于PHP的学生管理系统;
实现 登录、注册、学生信息、修改学生、删除学生、查询学生、添加学生等功能 ;
环境准备
开发平台:PhpStrom2022.1.2 、Phpstudy_pro
数据库:MySQL5.7.26
技术架构
Bootstrap +PHP7.3.4+html5+css3
项目结构
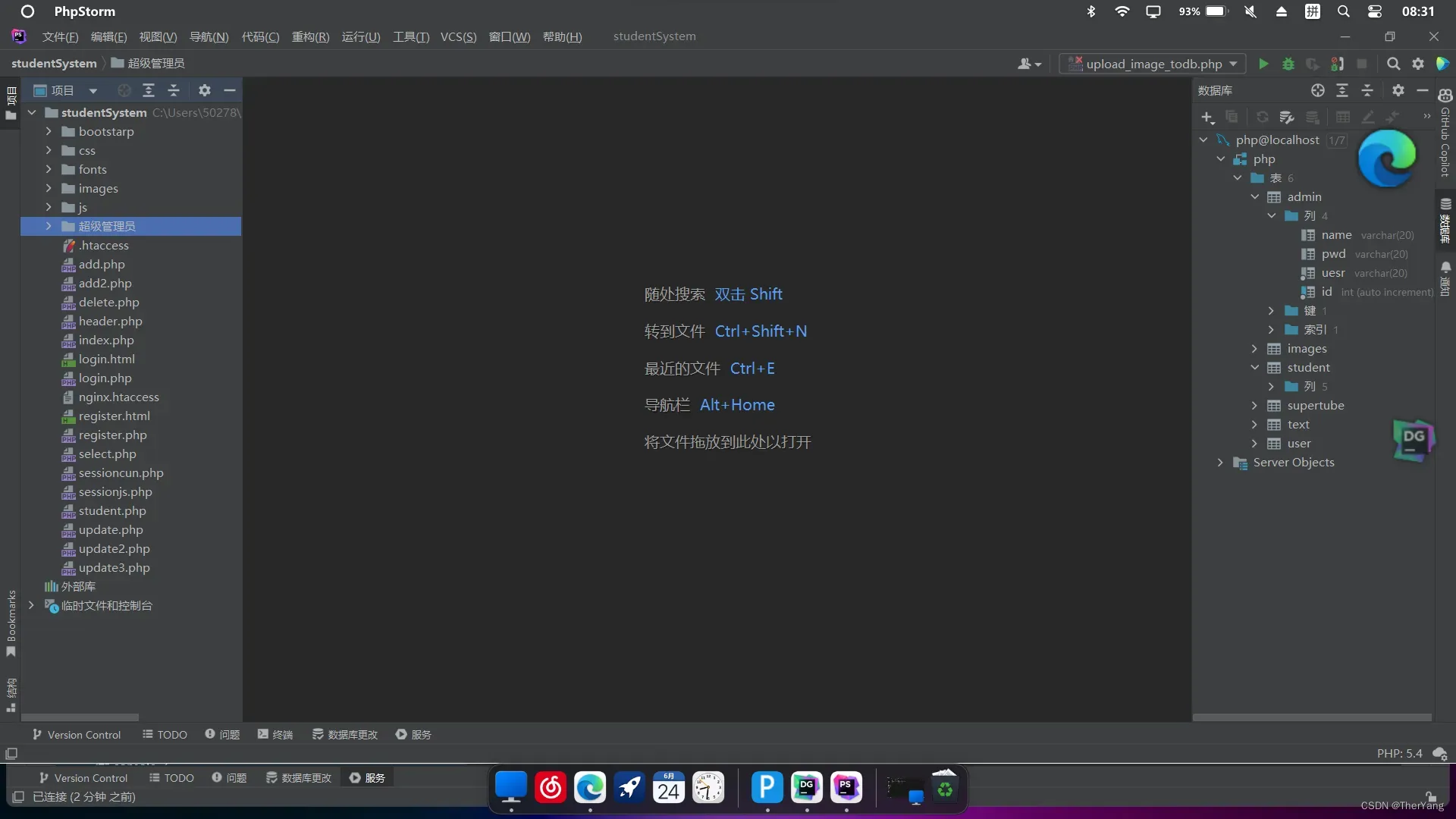
页面展示
1.登录与注册页面
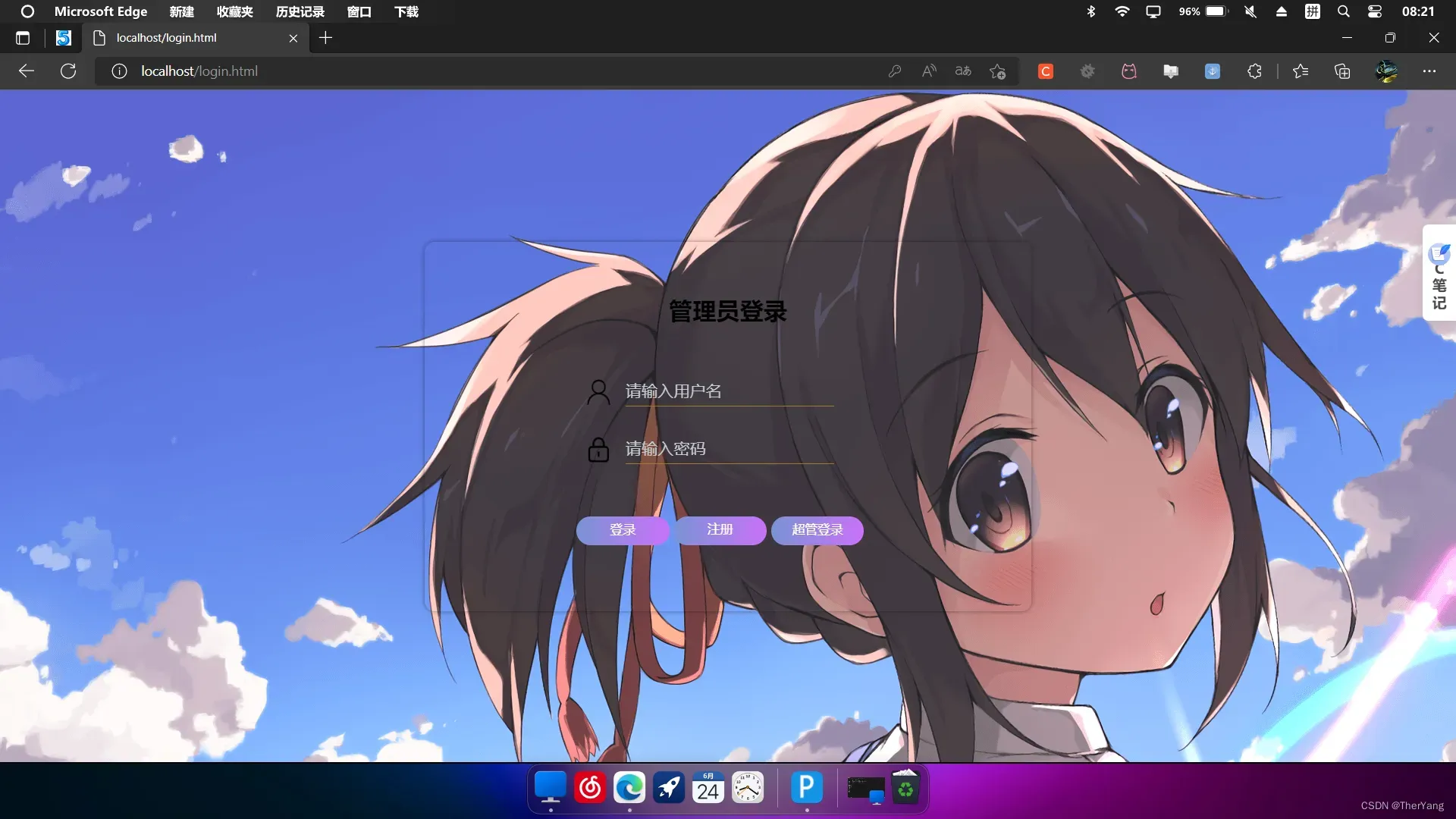
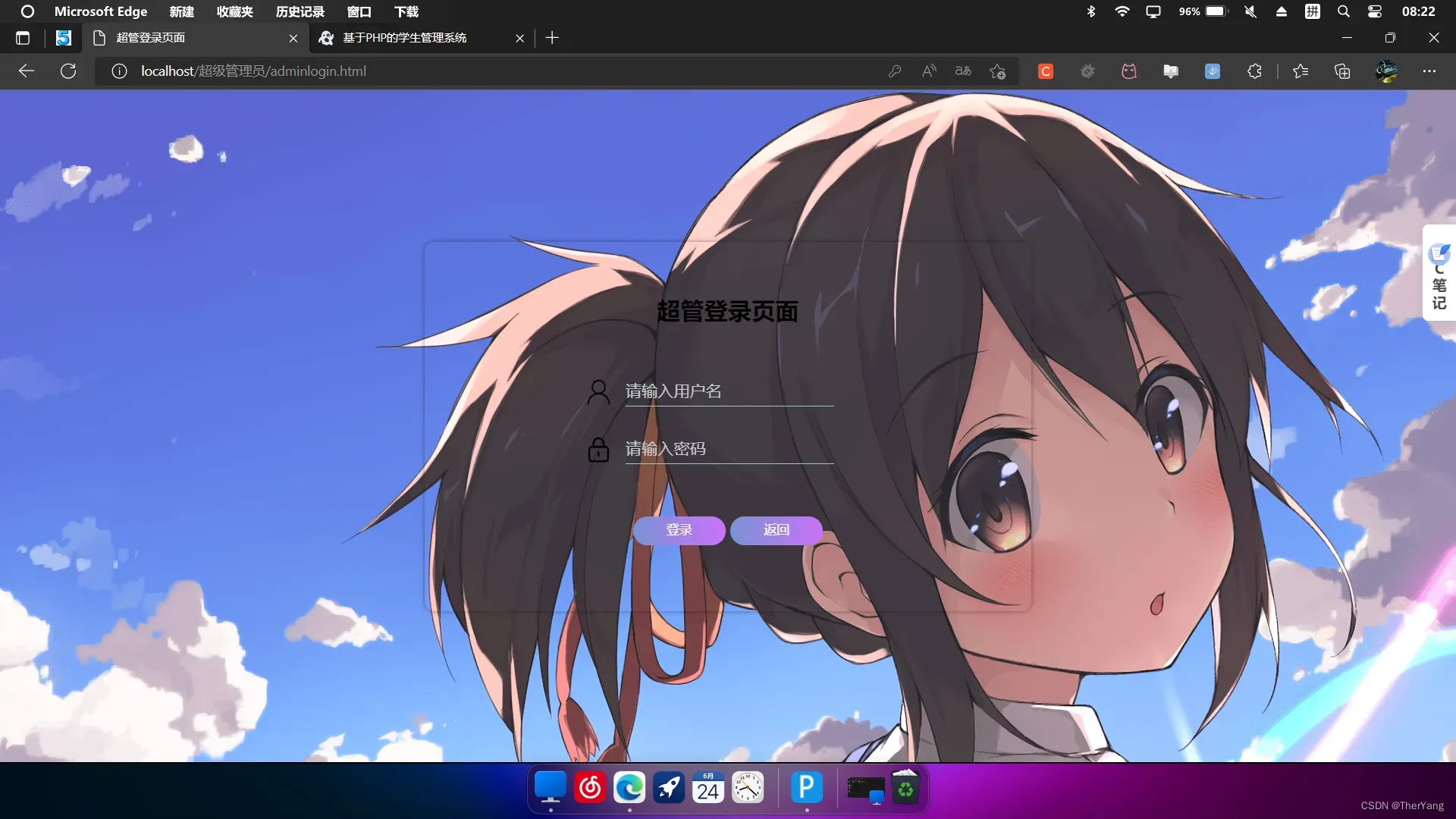
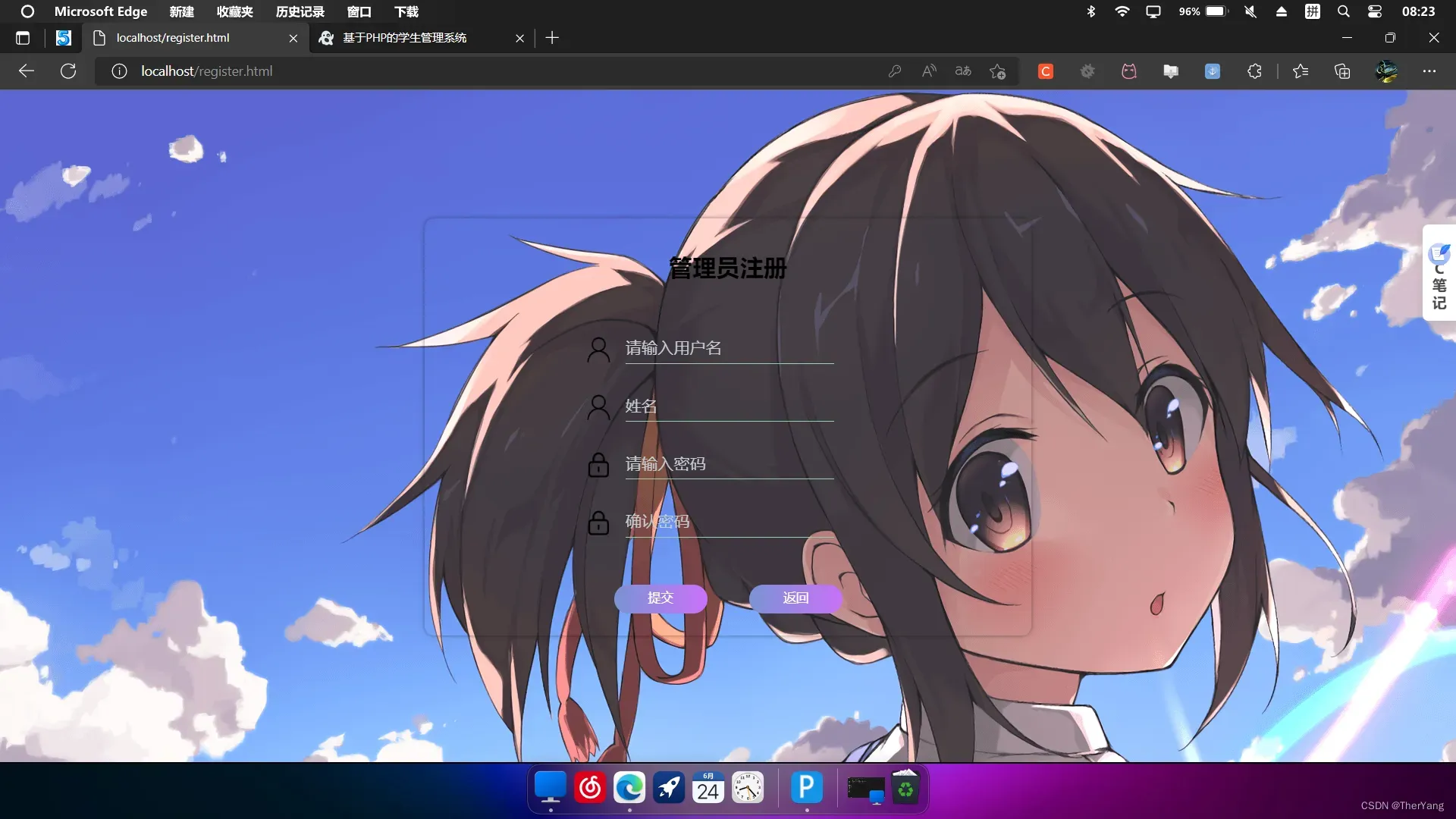
2.首页
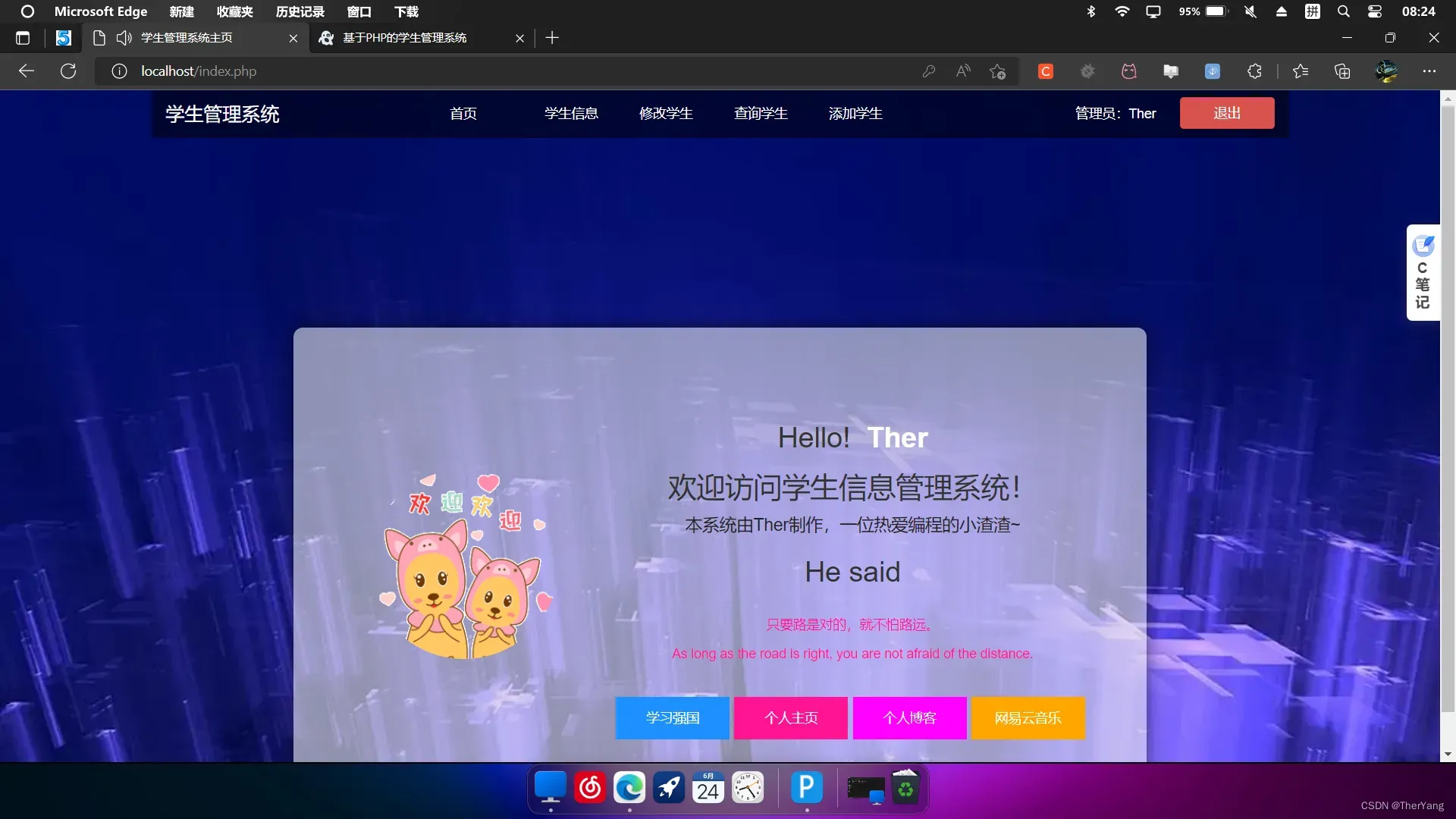
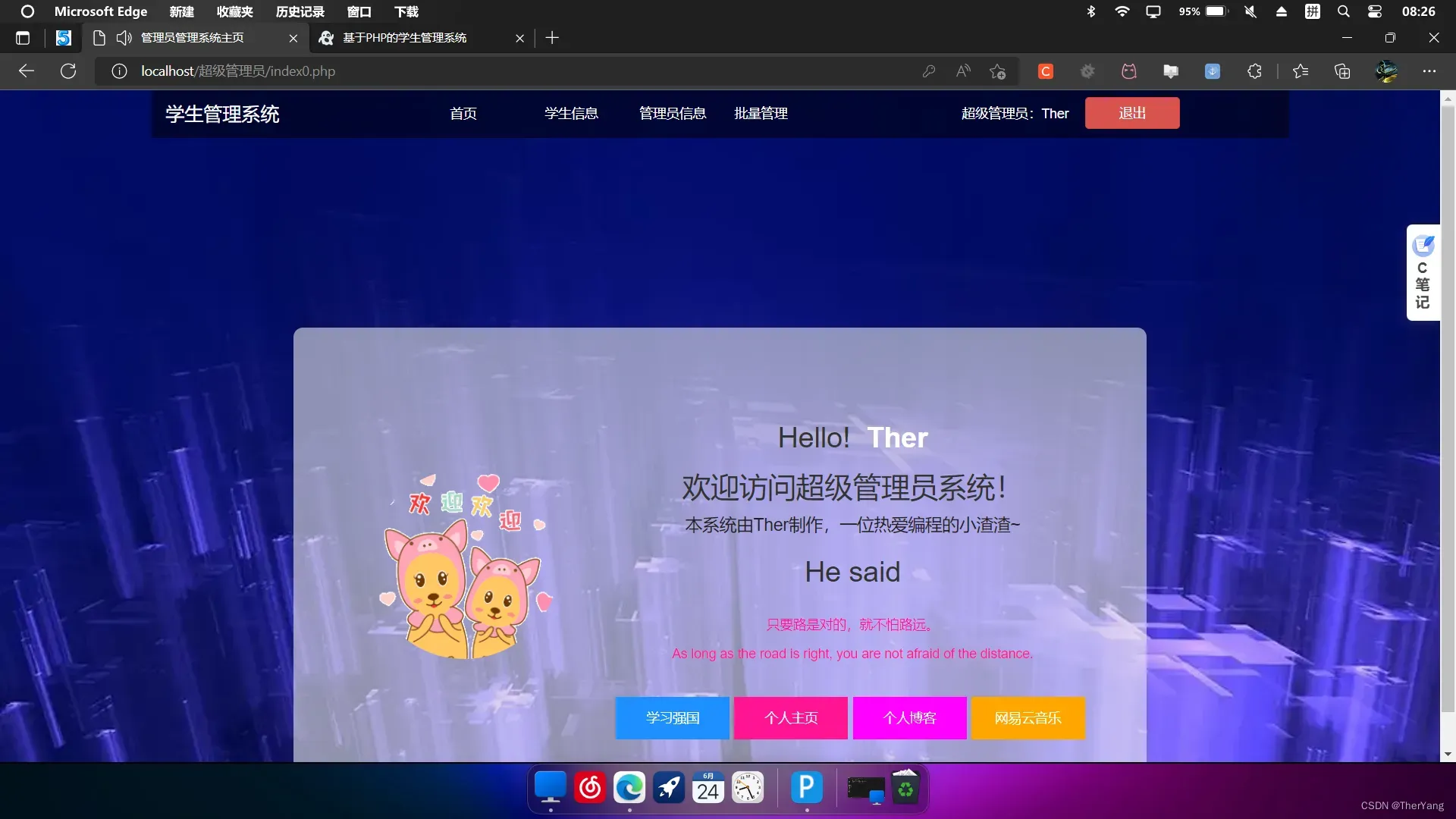
3.学生信息
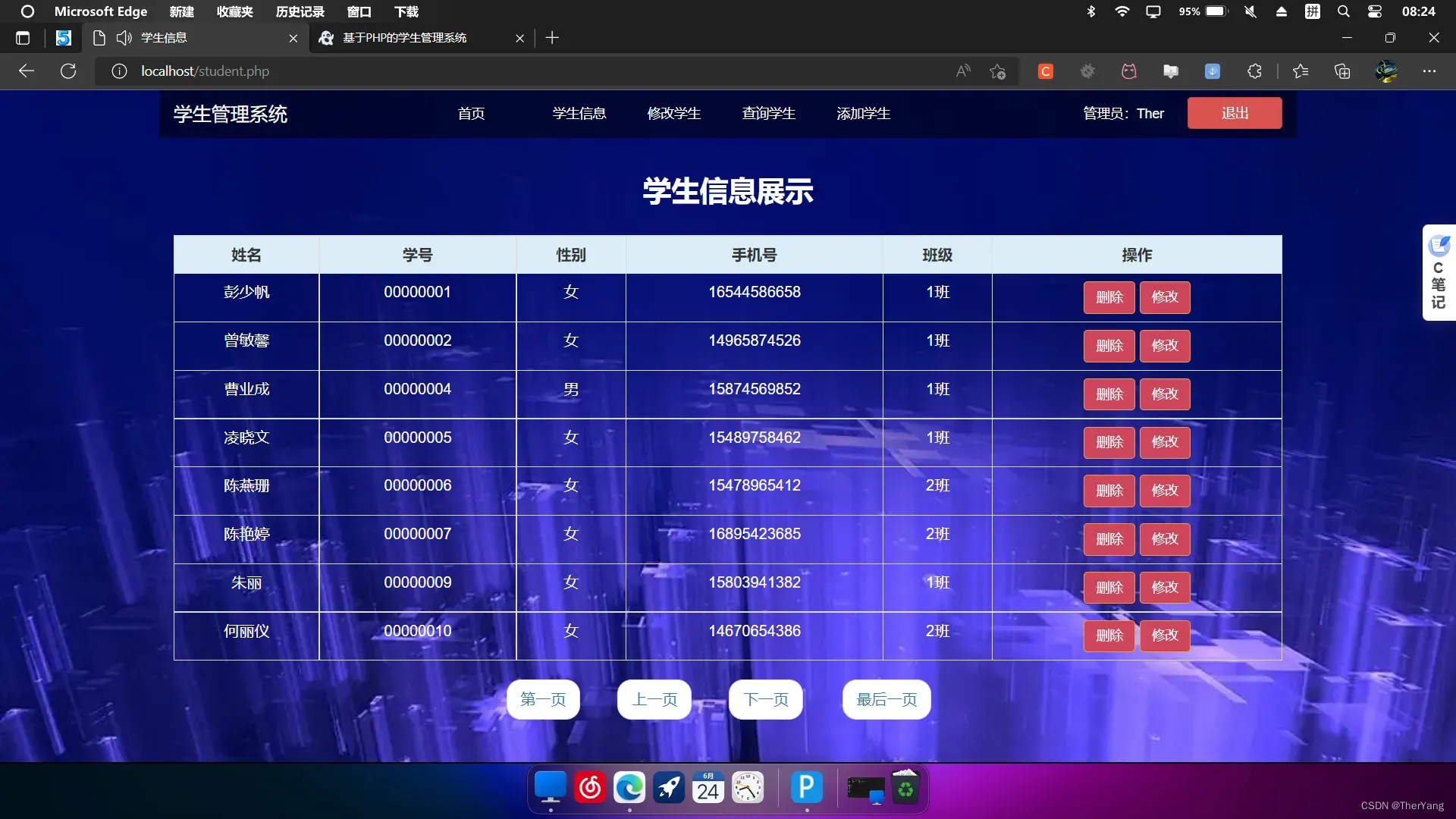
4.修改学生
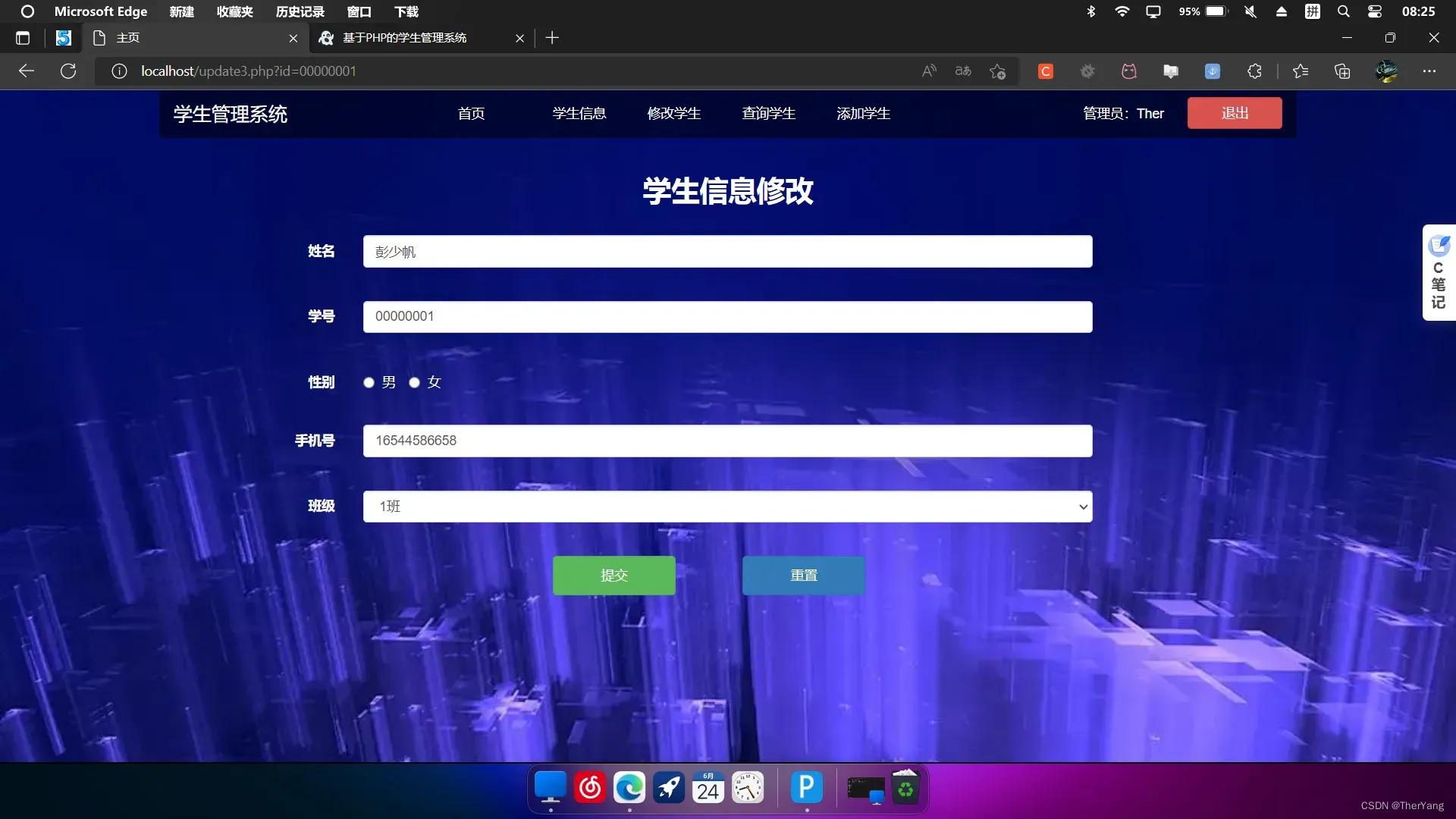
5.查询学生
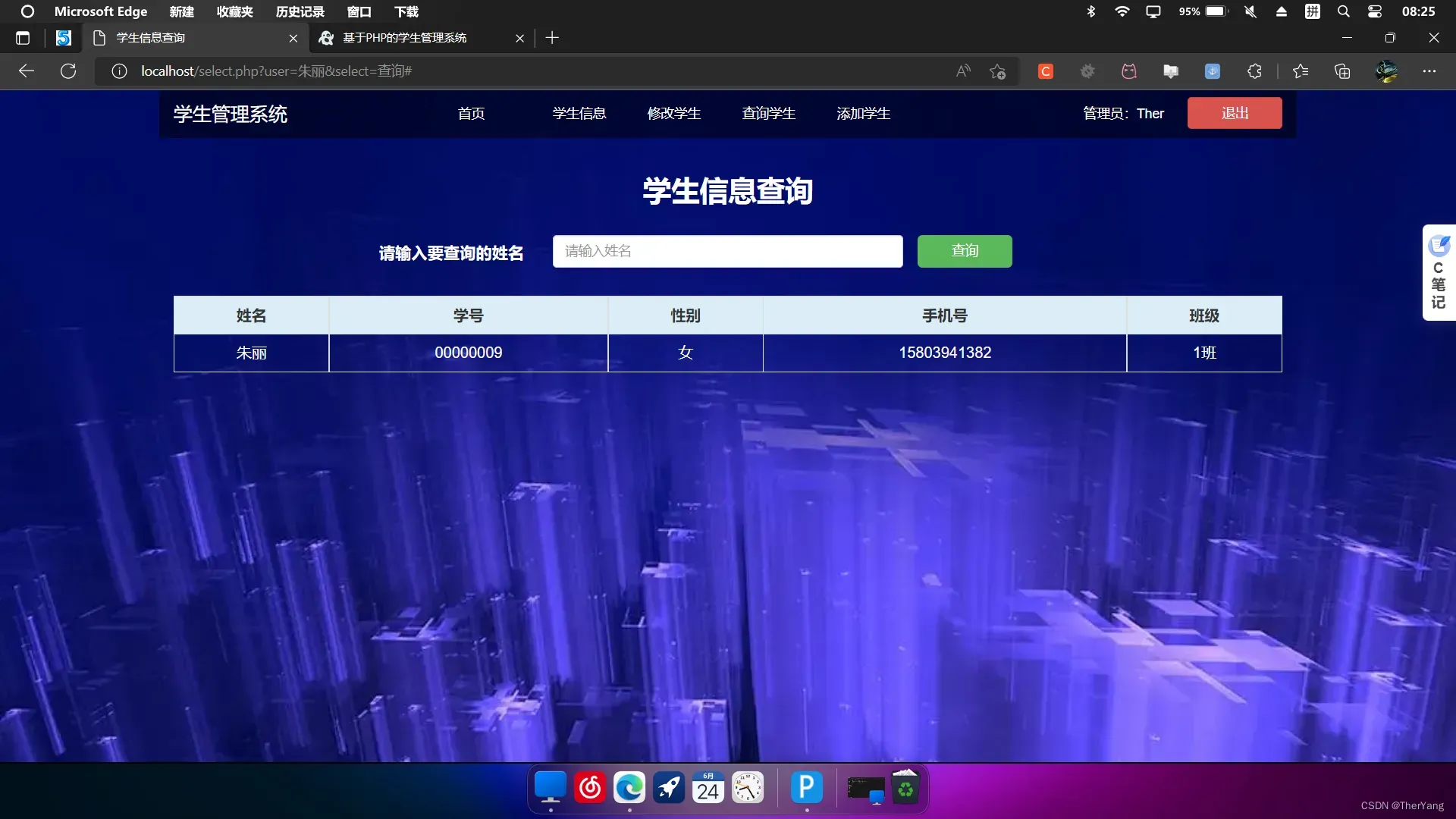
6.添加学生
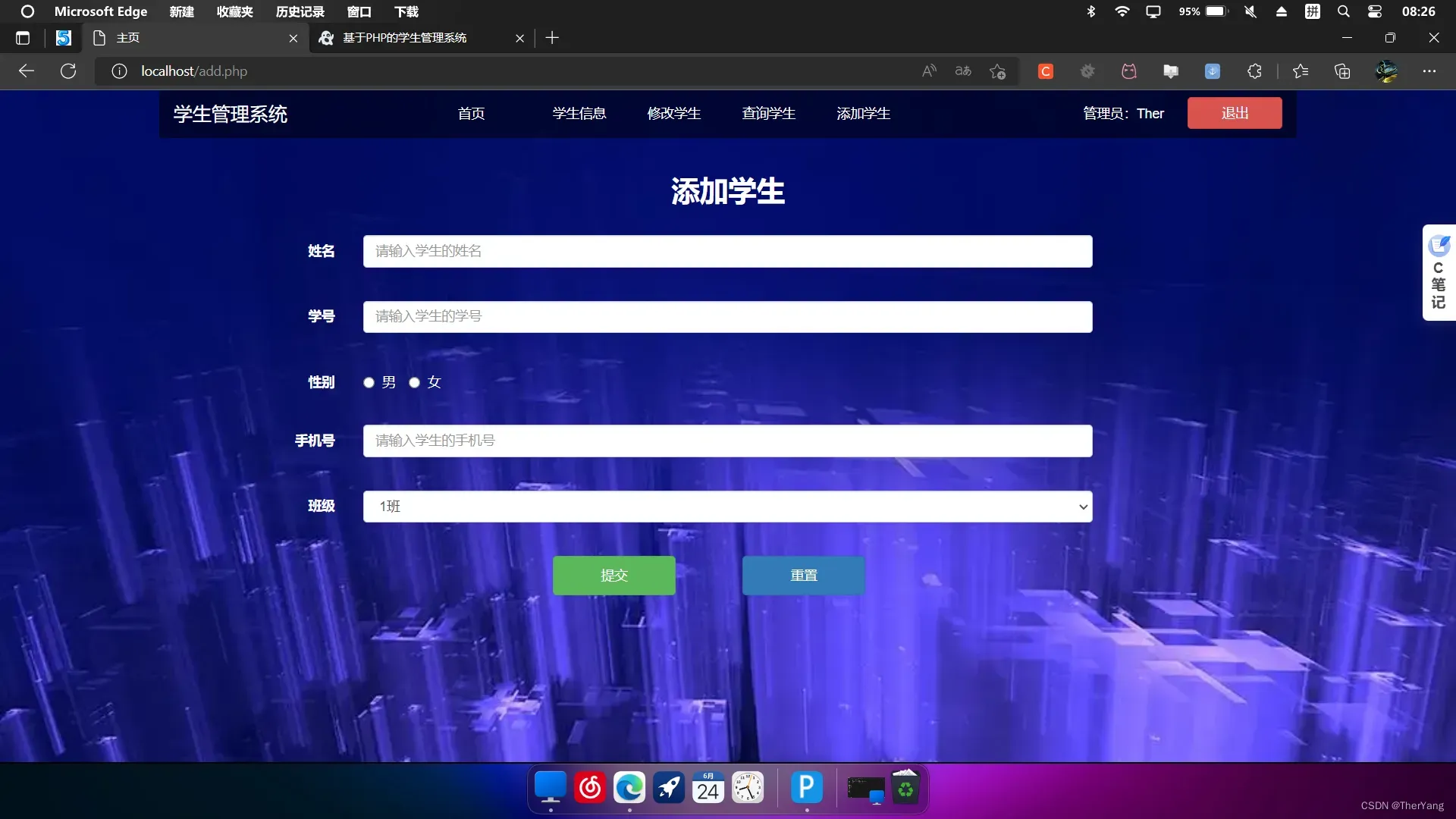
7.管理员信息
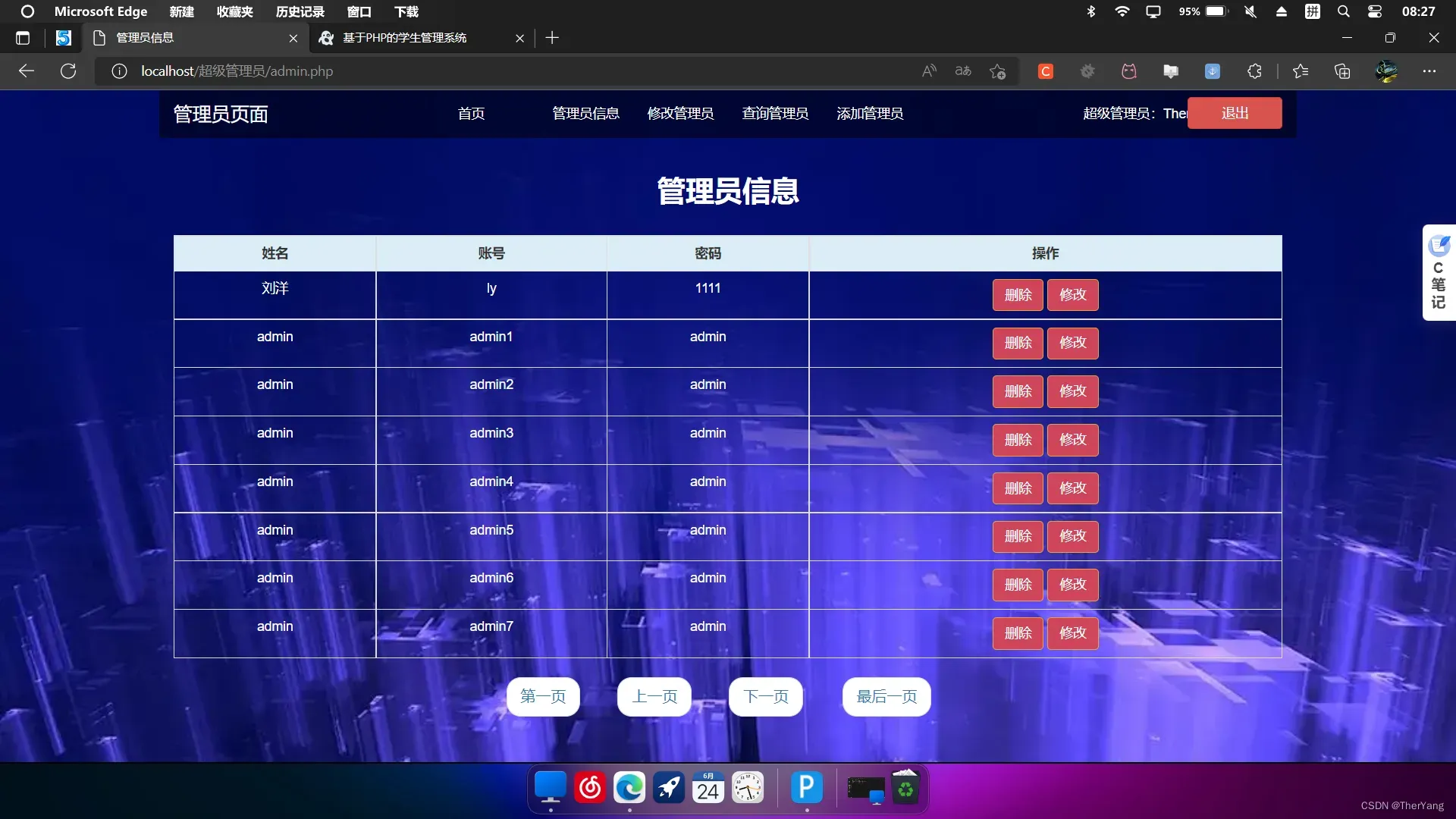
8.修改管理员
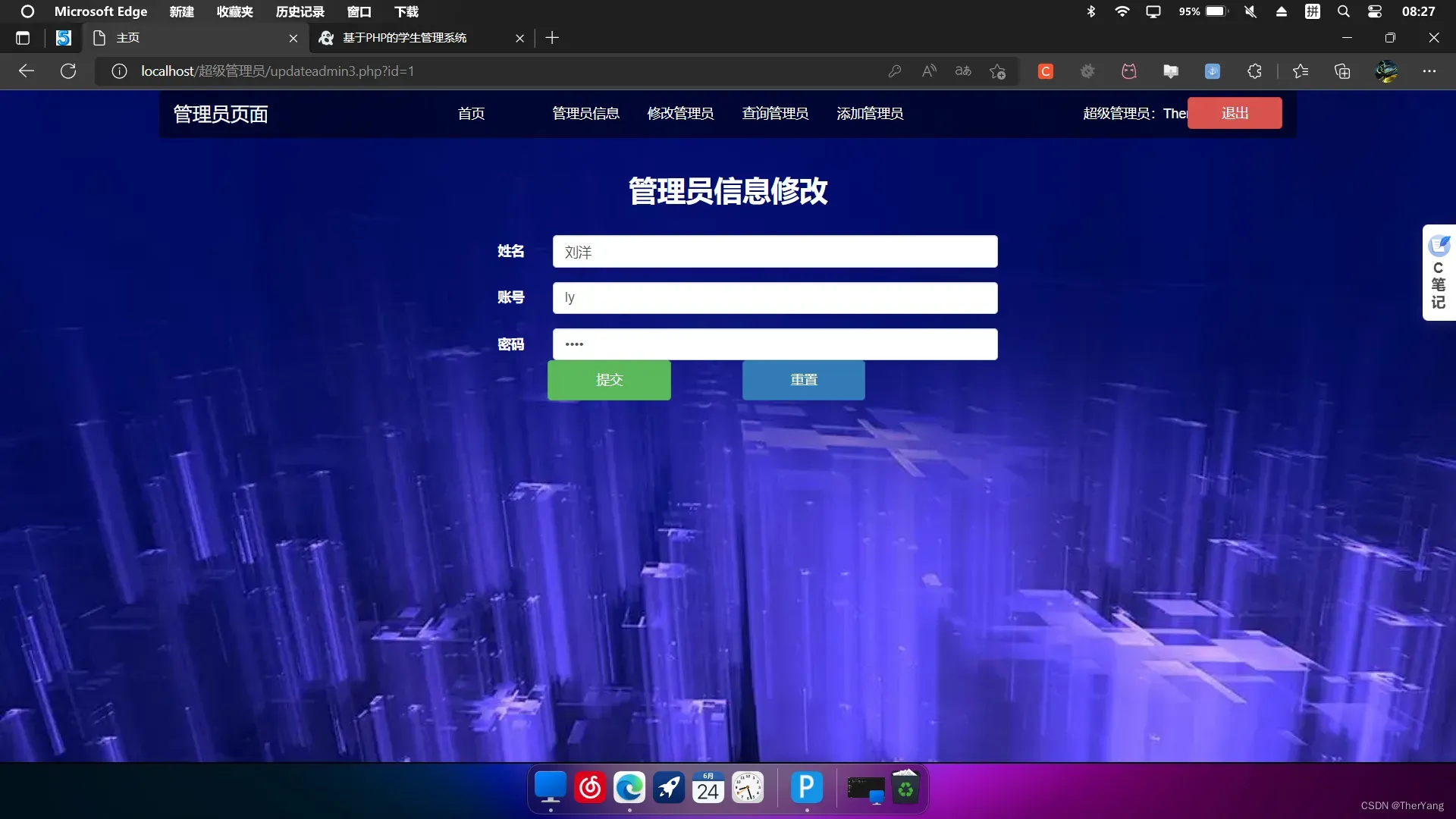
9.添加管理员
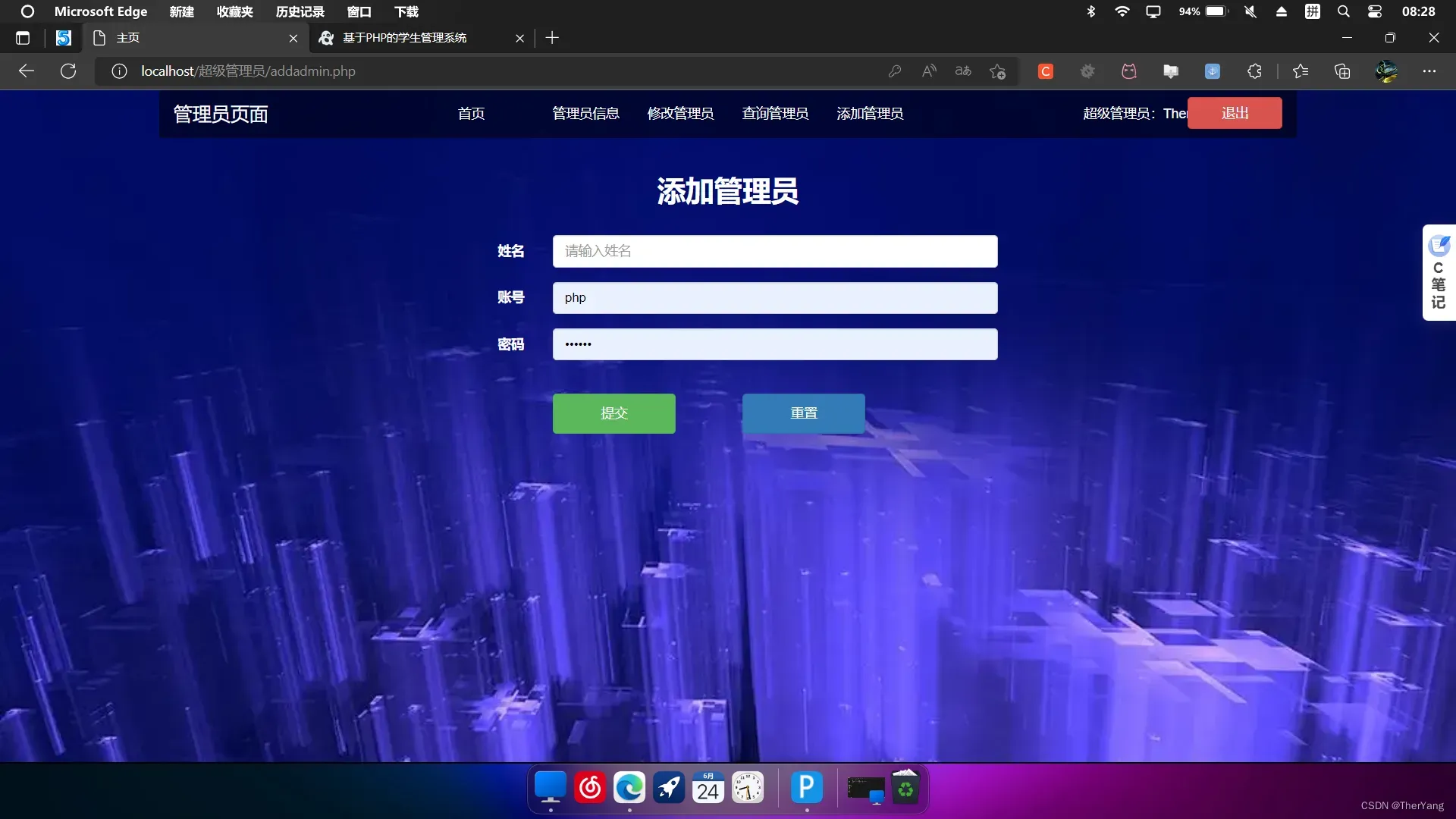
10.查询管理员
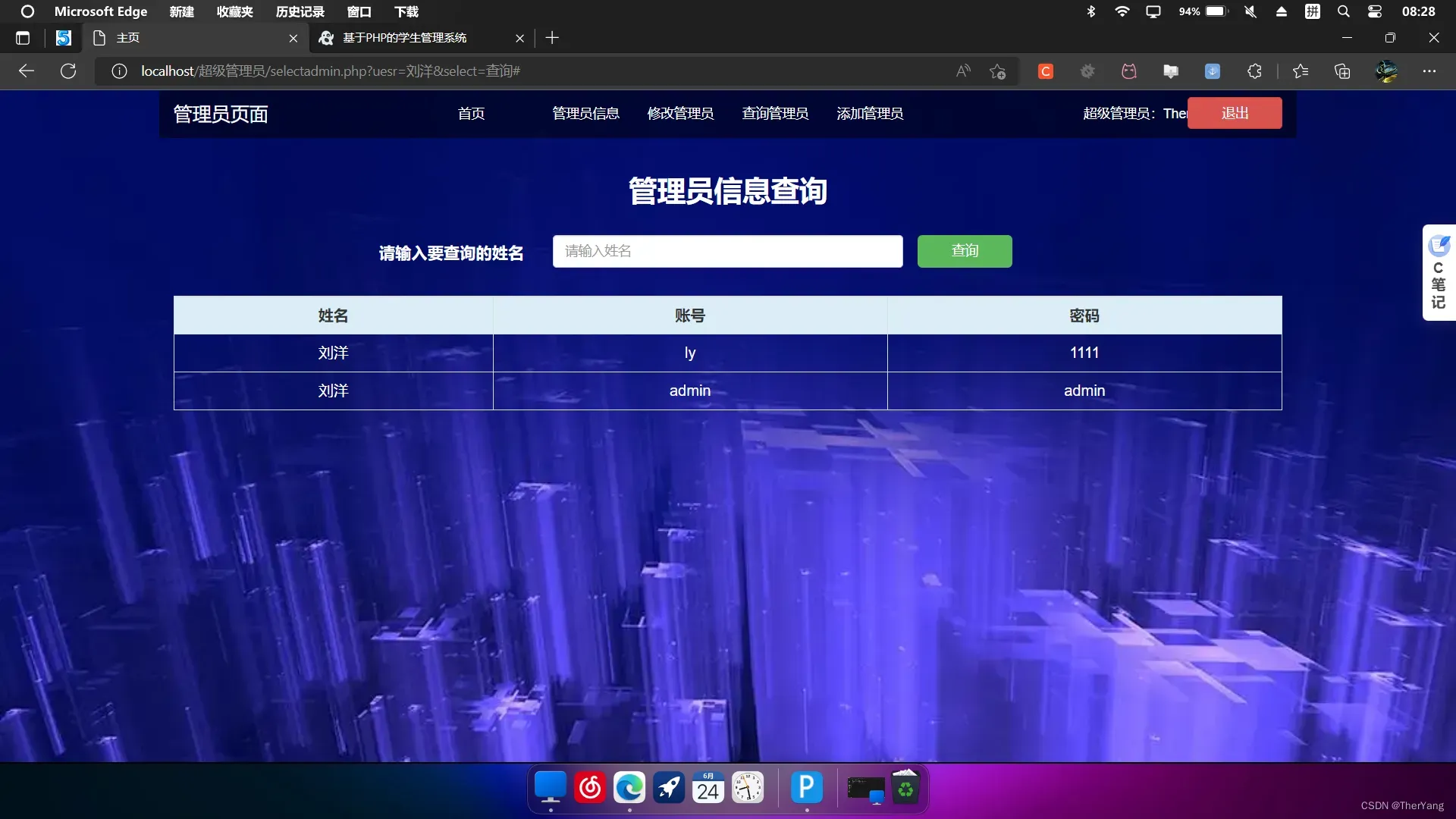
11.数据库
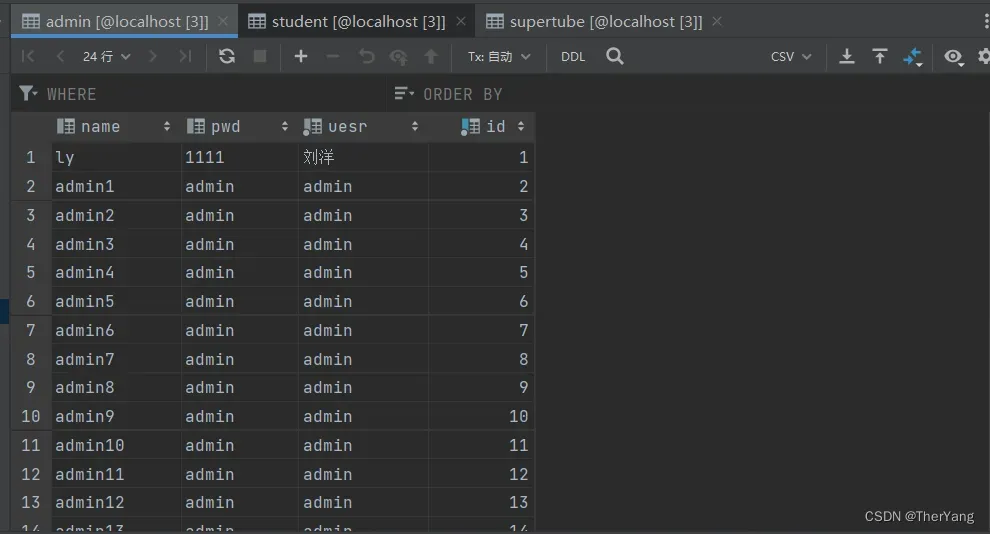
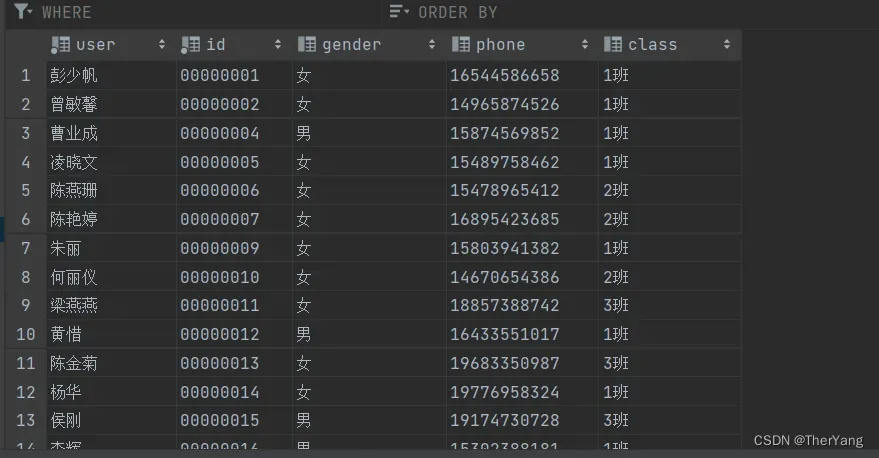
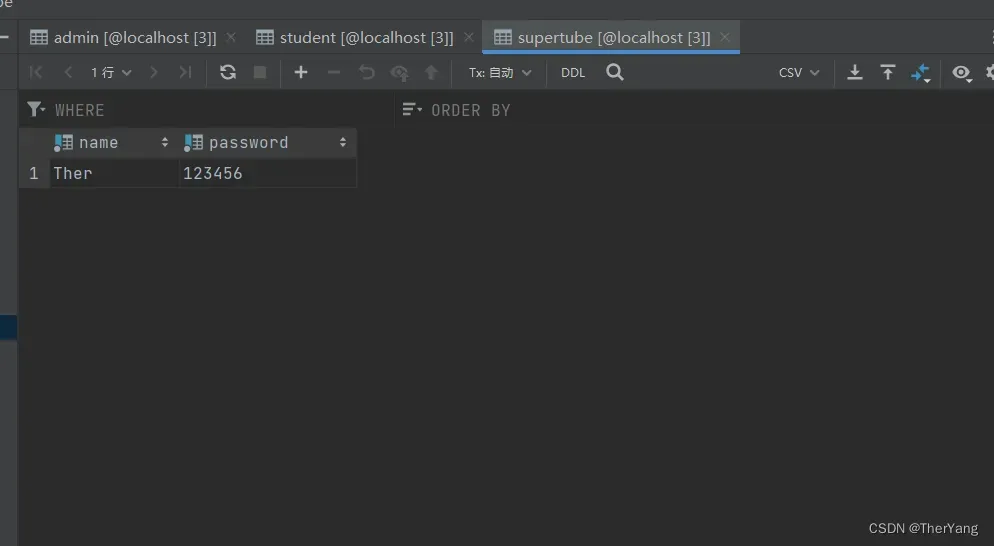
注意
1.MySQL 版本5.0以上与8.0以上sql语句有差异,需要调整数据库语句
2.运行项目前提前配置好MySQL数据库
3.本系统实现功能仅供参考
学生信息管理统代码展示
目录
一、登录界面
二、注册页面
三、学生信息
四、添加页面
五、修改页面
六、删除
七、查询页面
八、头部代码
一、登录界面
login.html
login.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<style type="text/css">
* {
margin: 0;
padding: 0;
}
body {
background: url(images/backgroud.png);
background-repeat: no-repeat;
background-size: cover;
background-attachment: fixed;
}
.Login {
width: 600px;
height: 350px;
background: rgb(168, 196, 213);
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
margin: auto;
border-radius: 10px;
padding: 20px;
box-shadow: 0 0 5px rgba(0,0,0,0.4);
}
label {
display: block;
margin-left: 10px;
}
form {
margin: 25px 140px;
}
h2 {
margin-top: 35px;
text-align: center;
font-size: 25px;
color: #000000;
}
input {
width: 220px;
height: 30px;
background: transparent;
margin-top: 30px;
border: none;
border-bottom: 1px #a77a27 solid;
outline: none;
color: #000000;
font-size: 17px;
margin-left: 10px;
}
input::-webkit-input-placeholder {
color: #c3cdd0;
}
button {
width: 98px;
height: 30px;
border: none;
margin-top: 55px;
color: white;
text-align: center;line-height: 15px;
background-image: linear-gradient(to right, #7993d4, #ca71fa);
border-radius: 15px;
cursor: pointer;
}
button:hover {
box-shadow: 0 0 5px rgba(0,0,0,0.4) inset;
}
img{
width: 27px;
height: 27px;
position: relative;
top: 9px;
}
</style>
</head>
<body>
<div class="Login">
<h2 >学生管理系统</h2>
<form action="login.php" method="post">
<label>
<img src="images/user.png">
<input type="text" name="user" placeholder="请输入用户名" required>
</label>
<label>
<img src="images/password.png">
<input type="password" name="pwd" placeholder="请输入密码" required>
</label>
<button type="submit" value="登录">登录</button>
<button type="button" id="btn" value="注册">注册</button>
<button type="button" id="btn0" value="超级管理员">超管登录</button>
</form>
</div>
<script>
var b = document.getElementById("btn");
b.onclick = function () {
window.location.href="register.html"
}
var b0 = document.getElementById("btn0");
b0.onclick = function () {
window.location.href="超级管理员/adminlogin.html"
}
</script>
</body>
</html>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<?php
header("Content-Type:text/html;charset=utf-8");
session_start();
//连接数据库
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
//设置编码
$conn->query("set names utf8");
$user = $_POST['user'];
$pwd = $_POST['pwd'];
$sql = "SELECT * FROM `admin` WHERE `name` = '{$user}' and `pwd` = '{$pwd}' ";
$stmt= $conn->query($sql);
$result = mysqli_fetch_array($stmt);
if($stmt->num_rows > 0){
$_SESSION['user'] = $result[0];
echo "<script>alert(\"登录成功!\")</script>";
echo "<script>window.location.href='index.php'</script>";
}else{
echo "<script>alert(\"账号或密码错误,请重新输入!\")</script>";
echo "<script>window.location.href='login.html'</script>";
}
二、注册页面
register.html
register.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<style type="text/css">
* {
margin: 0;
padding: 0;
}
body {
background: url(images/backgroud.png) no-repeat;
}
.Login {
width: 600px;
height: 400px;
background: rgb(232, 236, 223);
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
margin: auto;
border-radius: 10px;
padding: 20px;
box-shadow: 0 0 5px rgba(0,0,0,0.4);
}
label {
display: block;
margin-left: 10px;
}
form {
margin: 25px 140px;
}
h2 {
margin-top: 35px;
text-align: center;
font-size: 25px;
color: #000000;
}
input {
width: 220px;
height: 30px;
background: transparent;
margin-top: 30px;
border: none;
border-bottom: 1px #a77a27 solid;
outline: none;
color: #000000;
font-size: 17px;
margin-left: 10px;
}
input::-webkit-input-placeholder {
color: #c3cdd0;
}
button {
width: 98px;
height: 30px;
border: none;
margin-top: 50px;
margin-left: 40px;
color: white;
text-align: center;
line-height: 15px;
background-image: linear-gradient(to right, #7993d4, #ca71fa);
border-radius: 15px;
cursor: pointer;
}
button:hover {
box-shadow: 0 0 5px rgba(0,0,0,0.4) inset;
}
span {
font-size: 15px;
}
#btn > a{
text-decoration: none;
}
img{
width: 27px;
height: 27px;
position: relative;
top: 9px;
}
</style>
</head>
<body>
<div class="Login">
<h2>管理员注册</h2>
<form action="register.php" method="post">
<label>
<img src="images/user.png">
<input type="text" class="put" name="user" placeholder="请输入用户名" required>
</label>
<label>
<img src="images/password.png">
<input type="password" class="put" name="pwd1" placeholder="请输入密码" required>
</label>
<label>
<img src="images/password.png">
<input type="password" class="put" name="pwd2" placeholder="确认密码" required>
</label>
<button type="submit" value="提交">提交</button>
<button type="button" id="btn" value="返回">返回</button>
</form>
</div>
<script>
var b = document.getElementById("btn");
b.onclick = function () {
window.location.href="login.html"
}
</script>
</body>
</html>
<?php
session_start();
header("Content-Type:text/html;charset=utf-8");
//连接数据库
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
//设置编码
$conn->query("set names utf8");
$user = $_POST['user'];
$pwd1 = $_POST['pwd1'];
$pwd2 = $_POST['pwd2'];
$rows = "select `name` from `admin` where `name` = '{$user}'";
$stmt1= $conn->query($rows);
$row = mysqli_num_rows($stmt1);
if ($row == 1){
echo "<script>alert('此用户已存在,请重新输入!')</script>";
echo ("<script>window.location.href='register.html'</script>");
}
elseif ($pwd1 == $pwd2){
$sql = "insert into `admin` (`name`, `pwd`) value ('{$user}','{$pwd1}')";
$conn->query($sql);
echo ("<script>alert('注册成功')</script>");
echo ("<script>window.location.href='login.html'</script>");
}
else{
echo "<script>alert('密码错误,请重新输入!')</script>";
echo ("<script>window.location.href='register.html'</script>");
}
$conn->close();
三、学生信息
student.php
<?php
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
?>
<html>
<head>
<title>学生信息</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<!-- <link href="css/css1.css" rel="stylesheet">-->
<link href="bootstarp/dist/css/bootstrap.min.css" rel="stylesheet">
<style>
.btn-warning{
background-color: #d0455a;
}
</style>
</head>
<body>
<?php include("header.php");?>
<div class="container">
<br>
<h2 style="text-align: center;color: #3db077">学生信息展示</h2>
<br>
<table class="table table-bordered table-hover" style="text-align: center">
<tr class="info">
<th>姓名</th>
<th>学号</th>
<th>性别</th>
<th>手机号</th>
<th>班级</th>
<th>操作</th>
</tr>
<?php
$sql = "select * from `student` order by `id`";
$result = mysqli_query($conn,$sql);
if (mysqli_num_rows($result)>0) { // 若表中有数据
$number = mysqli_num_rows($result); // 取得数据笔数
if(!isset($_GET['p']))
{$p=0;}
else {$p=$_GET['p'];}
$check = $p + 8; // 每页抓取 8 笔数据
for ($i = 0; $i < $number; $i++) {// 用来呈现多笔数据的循环
$stu = mysqli_fetch_array($result);
//选取第 $p 笔到 $check 笔数据
if ($i >= $p && $i < $check) {
echo "<tr>";
echo "<td>{$stu['user']}</td>";
echo "<td>{$stu['id']}</td>";
echo "<td>{$stu['gender']}</td>";
echo "<td>{$stu['phone']}</td>";
echo "<td>{$stu['class']}</td>";
echo "<td><a href='delete.php?id={$stu['id']}' class='btn btn-warning'>删除</a>
<a href='update3.php?id={$stu['id']}' class='btn btn-warning'>修改</a></td>";
echo "</tr>";
$j = $i+1;
}
}// for循环
}
?>
</table>
<ul class="row pager" style="font-size: 16px;line-height: 30px">
<li class="col-xs-2 col-xs-push-3">
<!--- 将 p 值设为 0, 让模块从第一笔数据开始 ---->
<a href="student.php?p=0">第一页</a>
</li>
<li class="col-xs-2 col-xs-push-2" style="margin-left: 20px">
<?php
if ($p>7) { // 判断是否有上一页
$last = (floor($p/8)*8)-8;
echo "<a href='student.php?p=$last'>上一页</a>";
}
else
echo "<a class='disabled'>上一页</a>";
?>
</li>
<li class="col-xs-2 col-xs-push-1" style="margin-left: 20px">
<?php
if ($i>7 and $number>$check) // 判断是否有下一页
echo "<a href='student.php?p=$j'>下一页</a>";
else
echo "<a class='disabled'>下一页</a>";
?>
</li>
<li class="col-xs-2" style="margin-left: 30px">
<?php
if ($i>7) // 判断目前呈现的笔数之后是否还有页面
{
// 取得最后一页的第一笔数据
$final = floor($number/8)*8;
echo "<a href='student.php?p=$final'>最后一页</a>";
}
else
echo "<a class='disabled'>最后一页</a>";
?>
</li>
</ul>
</div>
</body>
</html>
四、添加页面
add.php
add2.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>主页</title>
<link href="bootstarp/dist/css/bootstrap.min.css" rel="stylesheet">
<link href="css/css1.css" rel="stylesheet">
</head>
<body>
<?php include("header.php");?>
<div class="container">
<br>
<h2 style="text-align: center;color: #3db077">添加学生</h2>
<br>
<div>
<form action="add2.php" method="post" class="form-horizontal" role="form">
<div class="form-group">
<label for="name" class="control-label col-xs-2">姓名</label>
<div class="col-xs-8">
<input id="name" type="text" name="user" class="form-control" placeholder="请输入学生的姓名" required>
</div>
</div>
<br>
<div class="form-group">
<label for="id" class="control-label col-xs-2">学号</label>
<div class="col-xs-8">
<input id="id" type="text" name="id" class="form-control" placeholder="请输入学生的学号" required>
</div>
</div>
<br>
<div class="form-group">
<label class="control-label col-xs-2">性别</label>
<div class="col-xs-8">
<label class="radio-inline"><input type="radio" value="男" name="gender" required>男</label>
<label class="radio-inline"><input type="radio" value="女" name="gender" required>女</label>
</div>
</div>
<br>
<div class="form-group">
<label for="phone" class="control-label col-xs-2">手机号</label>
<div class="col-xs-8">
<input id="phone" type="text" name="phone" class="form-control" placeholder="请输入学生的手机号" required>
</div>
</div>
<br>
<div class="form-group">
<label for="class" class="control-label col-xs-2">班级</label>
<div class="col-xs-8">
<!-- <input id="class" type="text" name="class" class="form-control" placeholder="请输入学生的班级" required>-->
<select name="class" class="form-control">
<option value="1班">1班</option>
<option value="2班">2班</option>
<option value="3班">3班</option>
<option value="4班">4班</option>
<option value="5班">5班</option>
<option value="6班">6班</option>
<option value="7班">7班</option>
<option value="8班">8班</option>
<option value="9班">9班</option>
<option value="10班">10班</option>
</select>
</div>
</div>
<br>
<div class="form-group">
<div class="col-xs-2 col-xs-offset-4">
<input style="padding: 10px 50px" class="btn btn-success" type="submit" value="提交">
</div>
<div class="col-xs-2">
<input type="reset" style="padding: 10px 50px" class="btn btn-primary" value="重置">
</div>
</div>
</form>
</div>
</div>
</body>
</html>
<?php
session_start();
//中文编码
header("Content-Type:text/html;charset=utf-8");
//连接数据库
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
//设置编码
$conn->query("set names utf8");
$user = $_POST['user'];
$id = $_POST['id'];
$gender = $_POST['gender'];
$phone = $_POST['phone'];
$class = $_POST['class'];
$rows = "select `id` from `student` where `id` = '{$id}'";
$stmt1= $conn->query($rows);
$row = mysqli_num_rows($stmt1);
if ($row == 1){
echo "<script>alert('此学号已存在,请重新输入!')</script>";
echo ("<script>window.location.href='add.php'</script>");
}else{
$sql = "insert into `student` (`user`,`id`,`gender`,`phone`,`class`) value ('{$user}','{$id}','{$gender}','{$phone}','{$class}')";
$stmt= $conn->query($sql);
if ($stmt > 0){
echo ("<script>alert('添加成功')</script>");
echo ("<script>window.location.href='student.php'</script>");
}else {
echo ("<script>alert('添加失败')</script>");
echo ("<script>window.location.href='student.php'</script>");
}
}
$conn->close();
五、修改页面
update.php
update2.php
update3.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>主页</title>
<link href="bootstarp/dist/css/bootstrap.min.css" rel="stylesheet">
<link href="css/css1.css" rel="stylesheet">
</head>
<body>
<?php include("header.php");?>
<div class="container">
<br>
<h2 style="text-align: center;color: #3db077">学生信息修改</h2>
<br>
<div>
<form action="update2.php" method="post" class="form-horizontal" role="form">
<div class="form-group">
<label for="name" class="control-label col-xs-2">姓名</label>
<div class="col-xs-8">
<input id="name" type="text" name="user" class="form-control" placeholder="请输入学生的姓名" required>
</div>
</div>
<br>
<div class="form-group">
<label for="id" class="control-label col-xs-2">学号</label>
<div class="col-xs-8">
<input id="id" type="text" name="id" class="form-control" placeholder="请输入学生的学号" required>
</div>
</div>
<br>
<div class="form-group">
<label class="control-label col-xs-2">性别</label>
<div class="col-xs-8">
<label class="radio-inline"><input type="radio" value="男" name="gender" required>男</label>
<label class="radio-inline"><input type="radio" value="女" name="gender" required>女</label>
</div>
</div>
<br>
<div class="form-group">
<label for="phone" class="control-label col-xs-2">手机号</label>
<div class="col-xs-8">
<input id="phone" type="text" name="phone" class="form-control" placeholder="请输入学生的手机号" required>
</div>
</div>
<br>
<div class="form-group">
<label for="class" class="control-label col-xs-2">班级</label>
<div class="col-xs-8">
<!-- <input id="class" type="text" name="class" class="form-control" placeholder="请输入学生的班级" required>-->
<select name="class" class="form-control">
<option value="1">1班</option>
<option value="2">2班</option>
<option value="3">3班</option>
<option value="4">4班</option>
<option value="5">5班</option>
<option value="6">6班</option>
<option value="7">7班</option>
<option value="8">8班</option>
<option value="9">9班</option>
<option value="10">10班</option>
</select>
</div>
</div>
<br>
<div class="form-group">
<div class="col-xs-2 col-xs-offset-4">
<input style="padding: 10px 50px" class="btn btn-success" type="submit" value="提交">
</div>
<div class="col-xs-2">
<input type="reset" style="padding: 10px 50px" class="btn btn-primary" value="重置">
</div>
</div>
</form>
</div>
</div>
</body>
</html>
<?php
header("Content-Type:text/html;charset=utf-8");
//连接数据库
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
//设置编码
$conn->query("set names utf8");
$user = $_POST['user'];
$id = $_POST['id'];
$gender = $_POST['gender'];
$phone = $_POST['phone'];
$class = $_POST['class'];
$rows = "select `id` from `student` where `id` = '{$id}' and `user` = '{$user}'";
$stmt1= $conn->query($rows);
$row = mysqli_num_rows($stmt1);
if ($row != 1){
echo "<script>alert('学号不可改变,请重新输入!')</script>";
echo ("<script>window.location.href='student.php'</script>");
}else{
$sql = "update `student` set `user` = '{$user}',`gender` = '{$gender}',`phone` = '{$phone}',`class` = '{$class}' where `id` = '{$id}'";
$stmt= $conn->query($sql);
if ($stmt > 0){
echo ("<script>alert('修改成功')</script>");
echo ("<script>window.location.href='student.php'</script>");
}else {
echo ("<script>alert('修改失败')</script>");
echo ("<script>window.location.href='student.php'</script>");
}
}
$conn->close();
<?php
header("Content-Type:text/html;charset=utf-8");
//连接数据库
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
//设置编码
$conn->query("set names utf8");
$sql = "SELECT * FROM student WHERE id =".$_GET['id'];
$stmt = $conn->query($sql);//返回预处理对象
$stu = $stmt->fetch_array(MYSQLI_ASSOC);//返回结果集为数组
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>主页</title>
<link href="bootstarp/dist/css/bootstrap.min.css" rel="stylesheet">
<link href="css/css1.css" rel="stylesheet">
</head>
<body>
<?php include("header.php");?>
<div class="container">
<br>
<h2 style="text-align: center">学生信息修改</h2>
<br>
<div>
<form action="update2.php" method="post" class="form-horizontal" role="form">
<div class="form-group">
<label for="user" class="control-label col-xs-2">姓名</label>
<div class="col-xs-8">
<input id="user" type="text" name="user" class="form-control" value="<?php echo $stu['user']?>" placeholder="请输入学生的姓名" required>
</div>
</div>
<br>
<div class="form-group">
<label for="id" class="control-label col-xs-2">学号</label>
<div class="col-xs-8">
<input id="id" type="text" name="id" class="form-control" value="<?php echo $stu['id']?>" placeholder="请输入学生的学号" required>
</div>
</div>
<br>
<div class="form-group">
<label class="control-label col-xs-2">性别</label>
<div class="col-xs-8">
<label class="radio-inline"><input type="radio" value="男" name="gender" required>男</label>
<label class="radio-inline"><input type="radio" value="女" name="gender" required>女</label>
</div>
</div>
<br>
<div class="form-group">
<label for="phone" class="control-label col-xs-2">手机号</label>
<div class="col-xs-8">
<input id="phone" type="text" name="phone" class="form-control" value="<?php echo $stu['phone']?>" placeholder="请输入学生的手机号" required>
</div>
</div>
<br>
<div class="form-group">
<label for="class" class="control-label col-xs-2">班级</label>
<div class="col-xs-8">
<!-- <input id="class" type="text" name="class" class="form-control" value="--><?php //echo $stu['class']?><!--" placeholder="请输入学生的班级" required>-->
<select name="class" class="form-control" value="<?php echo $stu['class']?>" >
<option value="1班">1班</option>
<option value="2班">2班</option>
<option value="3班">3班</option>
<option value="4班">4班</option>
<option value="5班">5班</option>
<option value="6班">6班</option>
<option value="7班">7班</option>
<option value="8班">8班</option>
<option value="9班">9班</option>
<option value="10班">10班</option>
</select>
</div>
</div>
<br>
<div class="form-group">
<div class="col-xs-2 col-xs-offset-4">
<input style="padding: 10px 50px" class="btn btn-success" type="submit" value="提交">
</div>
<div class="col-xs-2">
<input type="reset" style="padding: 10px 50px" class="btn btn-primary" value="重置">
</div>
</div>
</form>
</div>
</div>
</body>
</html>
六、删除
delete.php
<?php
header("Content-Type:text/html;charset=utf-8");
//连接数据库
$conn = new mysqli("localhost", "root", "123456", "php",3316)or die("连接失败");
//设置编码
$conn->query("set names utf8");
$id = $_GET['id'];
echo $id;
$sql = "delete from `student` where `id` = '{$id}'";
$stmt= $conn->query($sql);
if ($stmt > 0){
echo "<script>alert('删除成功')</script>";
echo "<script>window.location.href='student.php'</script>";
}else {
echo ("<script>alert('删除失败')</script>");
echo ("<script>window.location.href='student.php'</script>");
}
$conn->close();
七、查询页面
select.php
<?php
$conn = new mysqli("localhost", "root", "123456", "php", 3316) or die("连接失败");
?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<link href="css/css1.css" rel="stylesheet">
<title>主页</title>
<link href="bootstarp/dist/css/bootstrap.min.css" rel="stylesheet">
<style type="text/css">
th {
text-align: center;
}
label {
font-size: 17px;
}
</style>
</head>
<?php include("header.php");?>
<div class="container">
<br>
<h2 style="text-align: center;color: #3db077">学生信息查询</h2>
<br>
<form method="get" action="#" class="form-horizontal" role="form">
<div class="form-group">
<label for="name" class="control-label col-xs-2 col-xs-offset-2">请输入要查询的姓名</label>
<div class="col-xs-4">
<input id="name" type="text" name="user" class="form-control" placeholder="请输入姓名">
</div>
<input style="padding: 7px 30px;border-radius: 5px;border: none" class="col-xs-1 btn-success" type="submit"
name="select" value="查询">
</div>
</form>
<table class="table table-bordered table-hover" style="margin-top: 30px; text-align: center">
<tr class="info">
<th>姓名</th>
<th>学号</th>
<th>性别</th>
<th>手机号</th>
<th>班级</th>
</tr>
<?php
//名字模糊查询
if (isset($_GET['select'])) {
$user = $_GET['user'];
$sql = "select * from student where student.user like '%$user%'";
$result = $conn->query($sql);
while ($row = $result->fetch_assoc()) {
echo "<tr>";
echo "<td>" . $row['user'] . "</td>";
echo "<td>" . $row['id'] . "</td>";
echo "<td>" . $row['gender'] . "</td>";
echo "<td>" . $row['phone'] . "</td>";
echo "<td>" . $row['class'] . "</td>";
echo "</tr>";
}
}
?>
</table>
</div>
</body>
</html>
八、头部代码
header.php
<?php
$conn = new mysqli("localhost", "root", "123456", "php", 3316) or die("连接失败");
session_start();
?>
<html>
<head>
<title>主页</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<link href="css/css1.css" rel="stylesheet">
<link href="bootstarp/dist/css/bootstrap.min.css" rel="stylesheet">
<style>
button {
top: 7px;
right: 15px;
}
th {
text-align: center;
}
.container {
width: 1200px;
}
.row {
line-height: 50px;
}
.btn-warning {
background-color: #d0455a;
}
body {
background-image: url("images/backgroud.png");
background-repeat: no-repeat;
background-size: cover;
background-attachment: fixed;
}
</style>
</head>
<body>
<div class="container">
<div class="row" style="background-color: #2e7ad5;color: white">
<div style="font-size: 20px" class="col-xs-3">学生管理系统</div>
<a href="index.php"><span class="col-xs-1">首页</span></a>
<a href="student.php"><span class="col-xs-1">学生信息</span></a>
<a href="update.php"><span class="col-xs-1">修改学生</span></a>
<a href="select.php"><span class="col-xs-1">查询学生</span></a>
<a href="add.php"><span class="col-xs-1">添加学生</span></a>
<span style="left: 60px" class="col-xs-2 col-xs-offset-1">管理员:<?php echo $_SESSION['user'] ?></span>
<a href="login.html">
<button class="col-xs-1 btn btn-danger">退出</button>
</a>
</div>
</div>
</body>
</html>
文章出处登录后可见!
已经登录?立即刷新